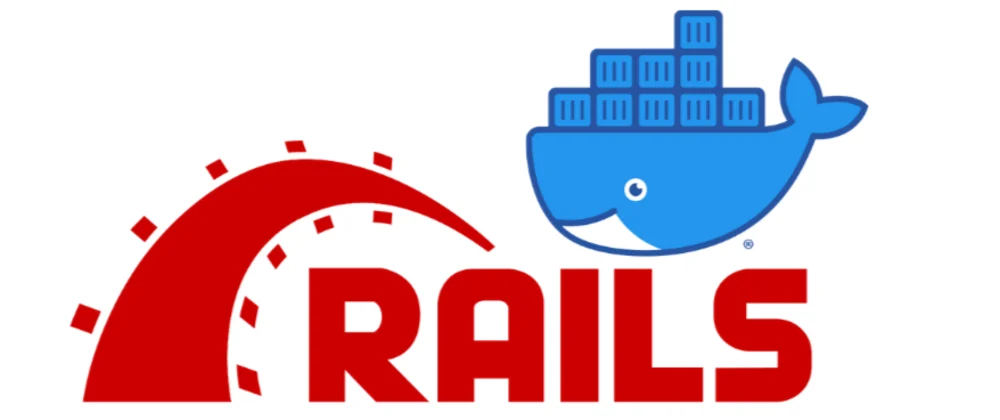
Problems?
What is your typical approach for creating a new Rails project?
When I first started learning Rails, I would install a new version of Ruby, followed by Rails, and then create a new project. However, this approach became tedious due to the need to manage Ruby dependencies for each project, dealing with version mismatches, and encountering various other issues.
As such, I sought out a way to streamline the process of creating new Rails projects. Docker has proven to be a reliable solution for this, enabling me to work efficiently and avoid many of the aforementioned challenges.
Steps
Before beginning, ensure that you have Docker installed on your computer. Next, follow these steps to create a new folder for your project:
$ mkdir my-new-rails-app
$ cd my-new-rails-app
Once inside the new folder, create a Dockerfile
with a minimal setup to support the desired Ruby version. You can find the image name corresponding to your Ruby version on Docker Hub. For example, if you’re using Ruby version 3.2.2, your Dockerfile should look like this:
FROM ruby:3.2.2
WORKDIR /usr/src/app
The WORKDIR
directive specifies the folder within the container that will host your code and will serve as the default location when accessing the container shell.
Next, create a docker-compose.yml
file with the following content:
services:
web:
build: .
ports:
- "3001:3000"
volumes:
- .:/usr/src/app
The 3001
port is used to access the server from the browser, while 3000
is the port inside the container.
The volume is essential because it allows the template files generated by the Rails application in the container to persist in the host filesystem.
Other information can be ignored at this stage
Now we can go ahead and access the terminal of the container while exposing the service ports so we can access the Rails application later on via localhost:3001:
docker-compose run --service-ports web bash
After running the above commands, you can install the Rails application inside the container using:
gem install rails
rails new .
Once you run these commands, take a look inside the my-new-rails-app
folder to see the entire Rails source.
Now you can have your Rails source without installing anything on your host machine.
Conclusion
Once you have the Rails source project, you can edit the Dockerfile and add more services like a database, etc. to complete the Rails environment setup.
This approach allows for easy creation of new Rails projects without worrying about rvm, rbenv or dependency overlap. I hope you found this article informative. Goodbye!