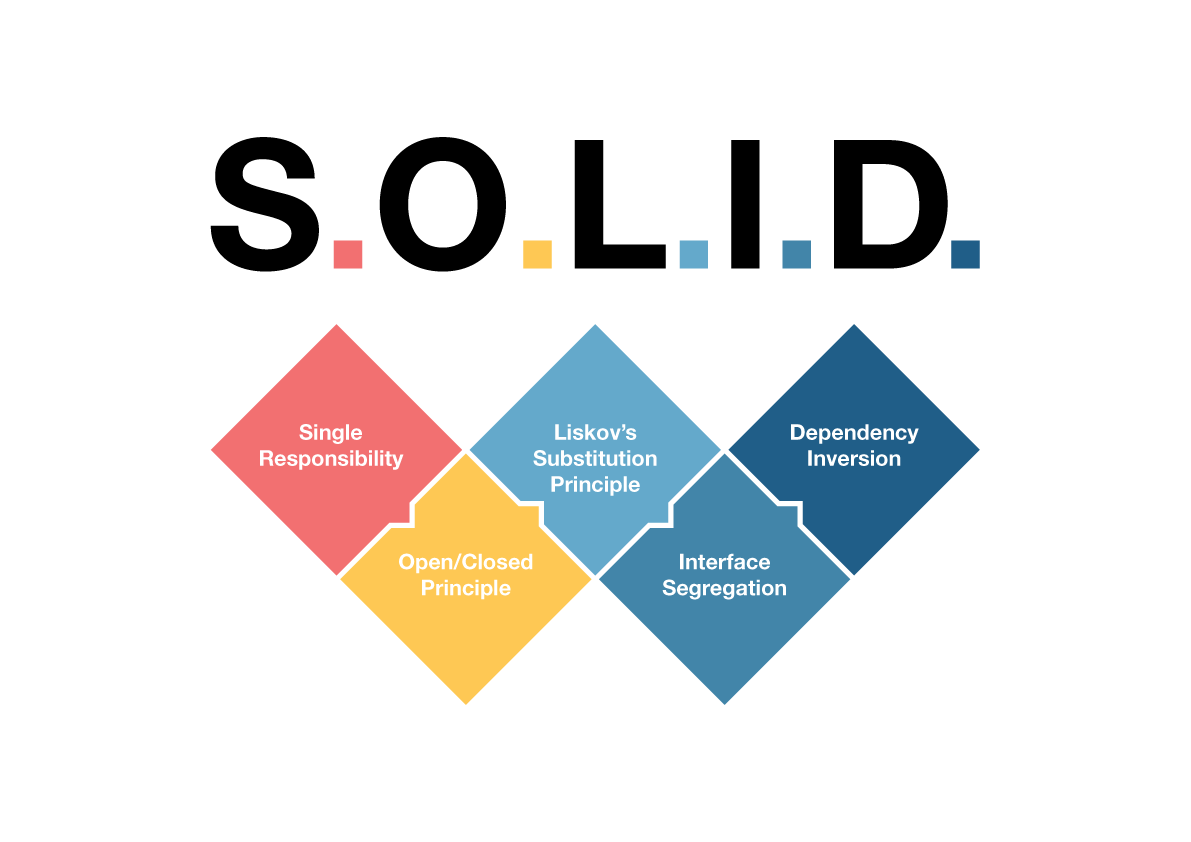
Understanding SOLID Principles in Ruby on Rails.
SOLID principles are a set of design guidelines that help developers create more maintainable, understandable, and flexible software. These principles are especially valuable in Ruby on Rails projects, where code can quickly become complex. In this blog post, we’ll explore each of the SOLID principles and provide examples of how you can apply them in your Rails applications.
S - Single Responsibility Principle (SRP)
The Single Responsibility Principle states that a class should have only one reason to change. This means that a class should only have one job or responsibility.
Example in Rails:
Suppose you have a User
model that handles user authentication and profile management. This violates SRP because the class has more than one responsibility. Let’s refactor it.
Before:
class User < ApplicationRecord
def authenticate(password)
# Authentication logic
end
def update_profile(params)
# Profile update logic
end
end
After:
class User < ApplicationRecord
end
class UserAuthenticator
def initialize(user)
@user = user
end
def authenticate(password)
# Authentication logic
end
end
class UserProfileUpdater
def initialize(user)
@user = user
end
def update_profile(params)
# Profile update logic
end
end
O - Open/Closed Principle (OCP)
The Open/Closed Principle states that software entities should be open for extension but closed for modification. This means you should be able to add new functionality without changing existing code.
Example in Rails:
Consider a Payment
class that processes different types of payments.
Before:
class Payment
def process(type)
if type == 'credit_card'
# Process credit card payment
elsif type == 'paypal'
# Process PayPal payment
end
end
end
After:
class Payment
def process(payment_method)
payment_method.process
end
end
class CreditCardPayment
def process
# Process credit card payment
end
end
class PayPalPayment
def process
# Process PayPal payment
end
end
L - Liskov Substitution Principle (LSP)
The Liskov Substitution Principle states that objects of a superclass should be replaceable with objects of a subclass without affecting the functionality of the program.
Example in Rails:
Imagine a Shape
class hierarchy where Square
and Rectangle
are subclasses.
Before:
class Rectangle
attr_accessor :width, :height
def initialize(width, height)
@width = width
@height = height
end
def area
@width * @height
end
end
class Square < Rectangle
def initialize(side)
@width = side
@height = side
end
end
After:
class Shape
def area
raise NotImplementedError, 'You must implement the area method'
end
end
class Rectangle < Shape
def initialize(width, height)
@width = width
@height = height
end
def area
@width * @height
end
end
class Square < Shape
def initialize(side)
@side = side
end
def area
@side * @side
end
end
I - Interface Segregation Principle (ISP)
The Interface Segregation Principle states that no client should be forced to depend on methods it does not use. This means creating specific interfaces for different clients.
Example in Rails:
Suppose you have a Report
class that generates different types of reports.
Before:
class Report
def generate_pdf
# Generate PDF report
end
def generate_csv
# Generate CSV report
end
end
After:
class Report
end
class PdfReport < Report
def generate
# Generate PDF report
end
end
class CsvReport < Report
def generate
# Generate CSV report
end
end
D - Dependency Inversion Principle (DIP)
The Dependency Inversion Principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions.
Example in Rails:
Consider a Notification
class that sends different types of notifications.
Before:
class Notification
def send_email(message)
# Send email notification
end
def send_sms(message)
# Send SMS notification
end
end
After:
class Notification
def initialize(sender)
@sender = sender
end
def send(message)
@sender.send(message)
end
end
class EmailSender
def send(message)
# Send email notification
end
end
class SmsSender
def send(message)
# Send SMS notification
end
end
Conclusion
Applying SOLID principles in your Ruby on Rails projects can significantly improve the maintainability and flexibility of your code. By following these guidelines, you can create software that is easier to understand, extend, and modify, ultimately leading to better and more robust applications.
Thôi Lo Code Đi Kẻo Sếp nạt!