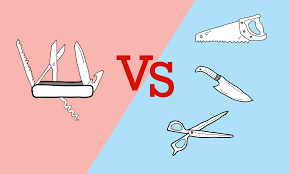
Understanding the Single Responsibility Principle with Real-World Examples
Hello, fellow software designers! Today, we’re diving into the Single Responsibility Principle (SRP), one of the five SOLID principles in software design. This principle helps create software components that are easier to manage, maintain, and extend by ensuring each component has a single, well-defined responsibility. Let’s explore this concept using a real-world analogy and then translate it into a practical software example.
What is the Single Responsibility Principle?
The Single Responsibility Principle (SRP) states that a class should have only one reason to change. In other words, a class should have only one job or responsibility. When a class takes on multiple responsibilities, it becomes harder to maintain, extend, and test.
Real-World Analogy: Restaurant Staff Roles
Let’s consider a restaurant to explain SRP with a real-world analogy:
- A restaurant has different staff roles: Chef, Waiter, and Cashier.
- Each role has a distinct responsibility:
- Chef: Responsible for preparing food.
- Waiter: Responsible for serving food to customers.
- Cashier: Responsible for handling payments.
Violation of SRP
Suppose in our restaurant, the Waiter is responsible not only for serving food but also for cooking and handling payments. This leads to several problems:
- Overloaded Responsibility: The waiter has too many roles, leading to confusion and inefficiency.
- Difficult to Maintain: Changes in one responsibility (like how payments are handled) could impact other responsibilities (like serving or cooking).
- Risk of Errors: The waiter might struggle to perform all these tasks effectively, leading to mistakes.
Correct Example Following SRP
Now, let’s assign each responsibility to a specific role:
- Chef: Prepares food.
- Waiter: Serves food to customers.
- Cashier: Handles payments.
By giving each role a single responsibility, we:
- Make each role easy to understand and manage.
- Improve efficiency by ensuring staff members focus on their specific tasks.
- Reduce the risk of errors and make restaurant operations smoother.
Translating SRP into Code
Now, let’s apply this concept to software design. We’ll create a program that represents the roles in the restaurant, ensuring each class has a single responsibility.
Implementing the Single Responsibility Principle in Ruby
We will define three classes: Chef
, Waiter
, and Cashier
. Each class will have a single responsibility aligned with the real-world example.
# Class responsible for preparing food
class Chef
def prepare_food(order)
"Preparing #{order}..."
end
end
# Class responsible for serving food
class Waiter
def serve_food(order)
"Serving #{order} to the customer."
end
end
# Class responsible for handling payments
class Cashier
def take_payment(amount)
"Processing payment of $#{amount}..."
end
end
Trade-Offs in Defining Responsibilities
When applying SRP, the challenge lies in finding the right balance for the size and scope of a class’s or component’s responsibility. Let’s explore both ends of the spectrum:
Trade-Offs of Too Large Responsibilities
- High Complexity: A class with multiple responsibilities becomes complex and harder to understand and maintain.
- Difficult to Modify: Changes in one responsibility can have unintended side effects on others.
- Poor Reusability: A class with multiple responsibilities is tightly coupled to its current context, making it hard to reuse.
Trade-Offs of Too Small Responsibilities
- Over-Engineering: Splitting responsibilities too much can lead to a proliferation of tiny classes that make the system harder to understand.
- Increased Complexity in Collaboration: Too many small classes require more interaction, increasing dependencies and coordination complexity.
- Performance Overhead: Having many small objects can lead to performance issues due to increased memory usage or the cost of frequent method calls.
How to Find the Right Balance
- Cohesion and Coupling: Aim for high cohesion within a class and low coupling between classes.
- Responsibility Granularity: Aim for a coherent grouping of responsibilities that makes sense in the business domain or application context.
- Context and Domain Knowledge: Use domain knowledge to determine what constitutes a “single responsibility.”
- Maintainability vs. Complexity Trade-Off: Strike a balance between maintainability and simplicity.
Conclusion
Finding the right level of granularity for a class’s responsibility requires careful consideration of trade-offs between maintainability and complexity. By understanding the context, leveraging domain knowledge, and considering both cohesion and coupling, you can determine the appropriate level of responsibility for each class or component in your design.
Remember, whether you’re designing a restaurant staff hierarchy or a software system, ensuring that each class or component has a single, well-defined responsibility leads to more manageable, flexible, and maintainable systems.
Stay tuned for more insights into software design principles and patterns!
Thôi Lo Code Đi Kẻo Sếp nạt!!