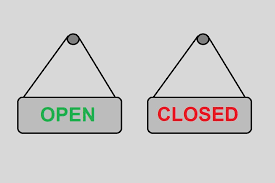
Understanding the Open/Closed Principle with Real-World Examples
Hello, fellow software designers! Today, we’re diving into the Open/Closed Principle (OCP), another key principle in the SOLID design principles. The OCP ensures that our software components are flexible and maintainable by being open for extension but closed for modification. Let’s explore this concept with a real-world analogy and then translate it into a practical software example.
What is the Open/Closed Principle?
The Open/Closed Principle (OCP) states that a class should be open for extension but closed for modification. This means you should be able to extend the behavior of a class without changing its existing code. By doing so, you minimize the risk of introducing bugs into existing functionality and promote flexibility and maintainability.
Real-World Analogy: Product Offers in a Store
Consider a store that offers different types of discounts and promotions on its products:
- Seasonal Discounts: Discounts that are available during specific seasons.
- Clearance Sales: Discounts on products that are being discontinued.
- Loyalty Discounts: Discounts for loyal customers based on their purchase history.
Violation of OCP
Imagine if the store had a single class, ProductOffer, responsible for handling all types of discounts and promotions:
class ProductOffer
def calculate_discount(product, type)
if type == "seasonal"
# Apply seasonal discount
elsif type == "clearance"
# Apply clearance discount
elsif type == "loyalty"
# Apply loyalty discount
else
# No discount
end
end
end
This implementation violates the Open/Closed Principle because:
- Modification Required for New Discounts: Whenever a new discount type is introduced (e.g., “Black Friday Discount”), the
ProductOffer
class must be modified to accommodate it. - High Risk of Bugs: Changes to the
calculate_discount
method can inadvertently affect existing discount logic, potentially introducing bugs. - Reduced Flexibility: The
ProductOffer
class is tightly coupled to all types of discounts, making it harder to maintain and extend.
Correct Example Following OCP
To adhere to the Open/Closed Principle, we should design the system so that new types of discounts can be added without modifying the existing ProductOffer
class. This can be achieved by using inheritance or composition.
Translating OCP into Code
Here’s how to implement the OCP correctly:
- Define a base class or interface, DiscountStrategy, with a method
calculate_discount
. - Create separate classes for each discount type, extending the base class and implementing the
calculate_discount
method.
# Base interface for discount strategies
class DiscountStrategy
def calculate_discount(product)
raise NotImplementedError, "Subclasses must implement the calculate_discount method"
end
end
# Seasonal discount strategy
class SeasonalDiscount < DiscountStrategy
def calculate_discount(product)
# Calculate seasonal discount
end
end
# Clearance sale discount strategy
class ClearanceDiscount < DiscountStrategy
def calculate_discount(product)
# Calculate clearance discount
end
end
# Loyalty discount strategy
class LoyaltyDiscount < DiscountStrategy
def calculate_discount(product)
# Calculate loyalty discount
end
end
# ProductOffer uses discount strategies
class ProductOffer
def initialize(discount_strategy)
@discount_strategy = discount_strategy
end
def apply_discount(product)
@discount_strategy.calculate_discount(product)
end
end
Explanation
- DiscountStrategy: An interface that defines the method
calculate_discount
, which each specific discount type must implement. - Specific Discount Classes:
SeasonalDiscount
,ClearanceDiscount
, andLoyaltyDiscount
are subclasses that provide their own implementations ofcalculate_discount
. - ProductOffer: Uses a discount strategy and does not need to be modified when new discount types are added. New discount strategies can be added by creating new subclasses that extend
DiscountStrategy
.
Benefits of Following OCP
- Flexibility: New discount types can be added easily without changing existing code.
- Maintainability: Existing code is not modified, reducing the risk of introducing bugs.
- Scalability: The system can easily scale to accommodate new features or business requirements.
Trade-Offs in Applying OCP
When applying the Open/Closed Principle, there are trade-offs to consider:
Trade-Offs of Not Following OCP
- Code Fragility: Frequent changes to existing classes can introduce bugs.
- Lack of Extensibility: It is difficult to add new functionality without modifying existing code.
- High Coupling: A single class may become too tightly coupled with different types of functionality.
Trade-Offs of Too Rigid Adherence to OCP
Over-Engineering: Creating too many small classes or strategies can lead to excessive complexity.
- Example: If you create a separate class for every possible type of discount, the codebase may become cluttered and harder to navigate.
Performance Considerations: Using many small classes may introduce a slight performance overhead, especially if there are many method calls or object creations.
Difficulty in Refactoring: Overuse of OCP might lead to difficulties when the business logic needs a substantial overhaul, as many classes would need to be changed or extended.
Finding the Right Balance
- Pragmatic Use of Inheritance and Composition: Use inheritance and composition thoughtfully to achieve a balance between flexibility and simplicity.
- Focus on Business Requirements: Align the design with real business needs. Avoid over-engineering by focusing on the most likely areas of change.
- Refactor When Necessary: Don’t hesitate to refactor code when requirements evolve. OCP does not mean avoiding all modifications but rather structuring the code to minimize them.
Conclusion
The Open/Closed Principle (OCP) helps create software that is easier to extend and maintain by encouraging designs that are open for extension but closed for modification. By applying OCP, you make your codebase more flexible, robust, and ready to accommodate future changes.
Whether you’re managing product offers in a store or developing complex software systems, adhering to OCP will help you build systems that are easier to adapt and grow with evolving business requirements.
Stay tuned for more insights into software design principles and patterns!
Thôi Lo Code Đi Kẻo Sếp nạt!!