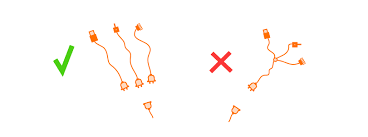
Understanding the Interface Segregation Principle with Real-World Examples
Hello, fellow software designers! Today, we’re exploring the Interface Segregation Principle (ISP), another crucial principle in the SOLID design principles. The ISP ensures that our software components remain modular, focused, and easy to maintain by providing clear and minimal interfaces. To illustrate this concept, we’ll use a real-world example and then apply it in a software context.
What is the Interface Segregation Principle?
The Interface Segregation Principle (ISP) states that “Clients should not be forced to depend on interfaces they do not use.” In simpler terms, an interface should be small and specific, containing only the methods that are relevant to its purpose. This prevents classes from being forced to implement methods they do not need.
Real-World Analogy: Service Staff in a Hotel
Let’s consider a hotel to explain the ISP with a real-world analogy:
- A hotel has different service staff roles: Housekeeper, Room Service, and Concierge.
- Each role has specific tasks:
- Housekeeper: Responsible for cleaning rooms.
- Room Service: Responsible for delivering food to guests.
- Concierge: Responsible for assisting guests with information and reservations.
Violation of ISP
Imagine if the hotel had a single interface called HotelServiceStaff that required all staff to implement methods for every possible task, like cleaning rooms, delivering food, and providing guest assistance:
# A bad example violating ISP
class HotelServiceStaff
def clean_room
raise NotImplementedError, "This method should be overridden by specific staff roles"
end
def deliver_food
raise NotImplementedError, "This method should be overridden by specific staff roles"
end
def provide_guest_assistance
raise NotImplementedError, "This method should be overridden by specific staff roles"
end
end
Here, every staff role (Housekeeper, Room Service, Concierge) is forced to implement methods they do not need:
- Housekeeper: Only needs
clean_room
, but is forced to implementdeliver_food
andprovide_guest_assistance
. - Room Service: Only needs
deliver_food
, but is forced to implementclean_room
andprovide_guest_assistance
. - Concierge: Only needs
provide_guest_assistance
, but is forced to implementclean_room
anddeliver_food
.
Problems with Violating ISP
- Unnecessary Implementations: Each role has to implement methods that are irrelevant to its actual responsibilities.
- Increased Maintenance: When the interface changes, all implementing classes must be modified, even if the change is not relevant to them.
- Reduced Clarity: The roles are less clear and focused, making the code harder to understand and maintain.
Correct Example Following ISP
To adhere to the Interface Segregation Principle, we should split the large HotelServiceStaff
interface into smaller, role-specific interfaces:
- Cleanable: For roles that need to clean rooms.
- FoodDeliverable: For roles that deliver food.
- GuestAssistanceProvidable: For roles that provide guest assistance.
Translating ISP into Code
Here’s how to implement the ISP correctly:
# Define small, specific interfaces for each role
module Cleanable
def clean_room
"Cleaning the room..."
end
end
module FoodDeliverable
def deliver_food
"Delivering food to the guest..."
end
end
module GuestAssistanceProvidable
def provide_guest_assistance
"Providing guest assistance..."
end
end
# Implement the interfaces with specific classes
class Housekeeper
include Cleanable
end
class RoomService
include FoodDeliverable
end
class Concierge
include GuestAssistanceProvidable
end
Benefits of Following ISP
- Focused Responsibilities: Each class implements only the methods it needs.
- Easier Maintenance: Changes in one interface do not affect classes that do not depend on that interface.
- Improved Clarity: Roles are clearly defined and easy to understand.
Trade-Offs in Defining Interfaces
When applying the ISP, the challenge is to find the right balance in defining interfaces:
Trade-Offs of Large Interfaces
- Unnecessary Implementations: Forcing classes to implement methods they do not need.
- High Coupling: Changes in the interface require all implementing classes to be updated.
- Poor Flexibility: Difficult to extend or modify functionality without affecting unrelated classes.
Trade-Offs of Too Small Interfaces
- Too Many Interfaces: Over-segmentation can lead to an excessive number of small interfaces, which can be hard to manage.
- Complexity in Client Code: Client code may need to work with multiple interfaces, increasing complexity.
- Performance Overhead: More interfaces might introduce slight performance overhead due to increased number of method calls.
How to Find the Right Balance
- Cohesion and Relevance: Group related methods together that are likely to change together.
- Understand Client Needs: Focus on the needs of the client classes that will use the interfaces.
- Context and Domain Knowledge: Leverage domain knowledge to define interfaces that represent meaningful abstractions.
Conclusion
The Interface Segregation Principle helps maintain a modular and flexible design by ensuring that classes are not forced to depend on methods they do not use. By splitting large interfaces into smaller, role-specific ones, we create a system that is easier to understand, maintain, and extend.
Remember, whether designing a hotel staff system or a software application, adhering to ISP leads to more robust, modular, and maintainable solutions.
Stay tuned for more insights into software design principles and patterns!
Thôi Lo Code Đi Kẻo Sếp nạt!!