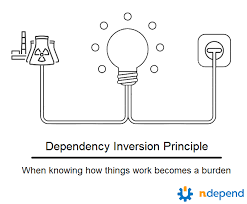
Understanding the Dependency Inversion Principle with Real-World Examples
Hello, fellow software designers! Today, we’re exploring the Dependency Inversion Principle (DIP), one of the foundational principles in the SOLID design principles. The DIP ensures that high-level modules are not tightly coupled to low-level modules, promoting flexibility and reducing the risk of changes rippling through your codebase. Let’s look at this principle through a real-world analogy and then translate it into a practical software example.
What is the Dependency Inversion Principle?
The Dependency Inversion Principle (DIP) states that:
- High-level modules should not depend on low-level modules. Both should depend on abstractions.
- Abstractions should not depend on details. Details should depend on abstractions.
In simpler terms, DIP encourages designing software so that high-level modules (business logic) do not depend directly on low-level modules (utility classes or APIs). Instead, both should rely on abstractions like interfaces or abstract classes, allowing the low-level details to change without affecting the high-level logic.
Real-World Analogy: Construction Project Management
Consider a construction project managed by a Project Manager. The manager oversees various tasks but does not directly control how each worker performs their job:
- Project Manager: Sets goals and coordinates the work.
- Electrician: Takes care of electrical installations.
- Plumber: Handles plumbing tasks.
- Carpenter: Manages woodwork and fittings.
Violation of DIP
Imagine if the Project Manager had to directly manage each worker’s detailed tasks:
ProjectManager:
- manageElectricalWork()
- managePlumbingWork()
- manageCarpentryWork()
In this setup:
- Tight Coupling: The Project Manager is tightly coupled with the specific details of electrical, plumbing, and carpentry work.
- Difficult to Change: If a new type of work is introduced (e.g., painting), the Project Manager must be modified to manage it.
- Low Flexibility: Changes in how a specific task is performed (e.g., a new method for electrical installations) require changes in the Project Manager.
Correct Example Following DIP
To adhere to the Dependency Inversion Principle, we should create an abstraction for the types of work:
- Worker Interface: An interface defining the general method of
perform_work
. - Specific Workers: Classes for
Electrician
,Plumber
,Carpenter
, etc., implementing theWorker
interface.
The Project Manager will depend on the Worker
interface, not on the specific worker classes.
Translating DIP into Code
Here’s how to implement the Dependency Inversion Principle correctly:
# Define the Worker interface
class Worker
def perform_work
raise NotImplementedError, "Subclasses must implement the perform_work method"
end
end
# Specific implementations of workers
class Electrician < Worker
def perform_work
"Performing electrical installations..."
end
end
class Plumber < Worker
def perform_work
"Handling plumbing tasks..."
end
end
class Carpenter < Worker
def perform_work
"Managing woodwork and fittings..."
end
end
# Project Manager depends on the abstraction (Worker interface)
class ProjectManager
def initialize(worker)
@worker = worker
end
def manage_work
@worker.perform_work
end
end
# Example usage
electrician = Electrician.new
manager = ProjectManager.new(electrician)
puts manager.manage_work # Output: "Performing electrical installations..."
Explanation
- Worker Interface: Defines a contract (
perform_work
) that all worker classes must fulfill. - Electrician, Plumber, Carpenter: Concrete implementations of the
Worker
interface. - ProjectManager: Depends on the
Worker
interface rather than concrete classes, allowing any worker to be managed without changing the manager’s code.
Benefits of Following DIP
- Flexibility: The high-level module (Project Manager) can work with any type of worker without needing modifications.
- Maintainability: Changes to low-level modules (workers) do not affect high-level modules.
- Testability: Makes it easier to substitute mock objects for testing purposes, as dependencies are on abstractions.
Trade-Offs in Applying DIP
When applying the Dependency Inversion Principle, there are trade-offs to consider:
Trade-Offs of Not Following DIP
- Tight Coupling: High-level modules become tightly coupled to low-level modules, making changes difficult and error-prone.
- Reduced Flexibility: Harder to adapt the system to new requirements or technologies.
- Low Reusability: Difficult to reuse components in different contexts since they depend on specific implementations.
Trade-Offs of Too Rigid Adherence to DIP
Over-Engineering: Introducing too many interfaces or abstractions can lead to overly complex code.
- Example: If you create an interface for every small detail, you might end up with too many unnecessary abstractions that complicate the design.
Performance Overhead: Excessive use of interfaces can introduce a slight performance cost due to the overhead of additional method calls or indirections.
Increased Complexity: Sometimes, too many layers of abstraction can make the system harder to understand and navigate.
Finding the Right Balance
- Use Abstractions Judiciously: Apply DIP where it provides clear benefits, like flexibility, testability, and maintainability.
- Avoid Over-Abstraction: Do not create interfaces for every small detail. Focus on the key areas where flexibility and decoupling are most needed.
- Keep the Domain in Mind: Understand the domain requirements to identify the right level of abstraction.
Conclusion
The Dependency Inversion Principle (DIP) helps create software that is more flexible, testable, and maintainable by decoupling high-level modules from low-level modules. By depending on abstractions rather than concrete implementations, you ensure that your code remains adaptable to future changes and requirements.
Whether managing a construction project or developing a complex software system, adhering to DIP will help you build robust, scalable, and maintainable solutions.
Stay tuned for more insights into software design principles and patterns!
Thôi Lo Code Đi Kẻo Sếp nạt!!