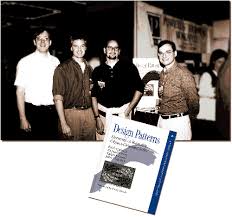
Overview of Design Patterns from GOF: The Foundations of Modern Software Design
Hey software designers! Today, we’re diving into the world of Design Patterns from the Gang of Four (GOF). These patterns have been the cornerstone of software design, offering tried-and-true solutions to common problems. Let’s explore their history, importance, real-world problems when not applying them, and the benefits of using them.
The History of GOF Design Patterns
The term “Design Patterns” in software development became widely recognized with the publication of the book “Design Patterns: Elements of Reusable Object-Oriented Software” in 1994 by Erich Gamma, Richard Helm, Ralph Johnson, and John Vlissides, collectively known as the Gang of Four (GOF). This seminal work was inspired by the earlier work of architect Christopher Alexander, who introduced the concept of design patterns in the context of building architecture.
The Importance of Design Patterns
Design patterns play a crucial role in software engineering for several reasons:
- Reusability: Patterns provide a template that can be reused in different contexts, reducing the need to reinvent solutions.
- Communication: They offer a common language for developers to discuss and convey complex design concepts.
- Best Practices: Patterns encapsulate best practices and proven solutions, helping to improve the quality and maintainability of software.
- Flexibility: By following patterns, developers can create systems that are more flexible and easier to modify as requirements change.
Problems Without Design Patterns
Imagine you’re building a house without a blueprint. You might end up with a structurally unsound building, rooms that don’t fit together well, and a lot of wasted materials. The same applies to software design. Without design patterns, you may face:
- Rigid Code: Hard-to-change code because dependencies are tightly coupled.
- Duplicate Code: Repetitive solutions scattered throughout the codebase, making maintenance a nightmare.
- Poor Scalability: Systems that can’t easily grow or adapt to new requirements.
- Difficult Debugging: Increased complexity and intertwined code, making it harder to identify and fix bugs.
Benefits of Applying Design Patterns
Now, let’s consider the benefits of applying design patterns with some real-world examples:
Creational Patterns
Example: Singleton Pattern
Without Singleton:
class Logger
def log(message)
# Log message
end
end
# Multiple instances can cause inconsistency
logger1 = Logger.new
logger2 = Logger.new
With Singleton:
class Logger
@@instance = Logger.new
def self.instance
@@instance
end
def log(message)
# Log message
end
private_class_method :new
end
# Consistent single instance
logger1 = Logger.instance
logger2 = Logger.instance
Benefits: Ensures a single instance of Logger
, providing consistent logging and saving resources.
Structural Patterns
Example: Adapter Pattern
Without Adapter:
class EuropeanPlug
def plug_in
# Plug in with European standards
end
end
class AmericanSocket
def plug_in
# Plug in with American standards
end
end
# Incompatible interfaces
european_plug = EuropeanPlug.new
american_socket = AmericanSocket.new
With Adapter:
class EuropeanPlugAdapter
def initialize(european_plug)
@european_plug = european_plug
end
def plug_in
@european_plug.plug_in
end
end
european_plug = EuropeanPlug.new
adapter = EuropeanPlugAdapter.new(european_plug)
adapter.plug_in
Benefits: Allows incompatible interfaces to work together, enhancing flexibility and reusability.
Behavioral Patterns
Example: Observer Pattern
Without Observer:
class DataSource
def initialize
@data = []
end
def add_data(data)
@data << data
# Notify all views (tightly coupled)
view1.update(data)
view2.update(data)
end
end
class View1
def update(data)
# Update view
end
end
class View2
def update(data)
# Update view
end
end
With Observer:
class DataSource
def initialize
@data = []
@observers = []
end
def add_observer(observer)
@observers << observer
end
def add_data(data)
@data << data
notify_observers(data)
end
private
def notify_observers(data)
@observers.each { |observer| observer.update(data) }
end
end
class Observer
def update(data)
# Update observer
end
end
view1 = Observer.new
view2 = Observer.new
data_source = DataSource.new
data_source.add_observer(view1)
data_source.add_observer(view2)
Benefits: Decouples the data source and observers, making the system more flexible and easier to maintain.
Conclusion
The design patterns introduced by the GOF have stood the test of time and continue to be relevant in modern software development. They offer a toolkit of solutions that can make your code more robust, flexible, and maintainable. Embrace these patterns and incorporate them into your projects to become a more effective and efficient developer.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!