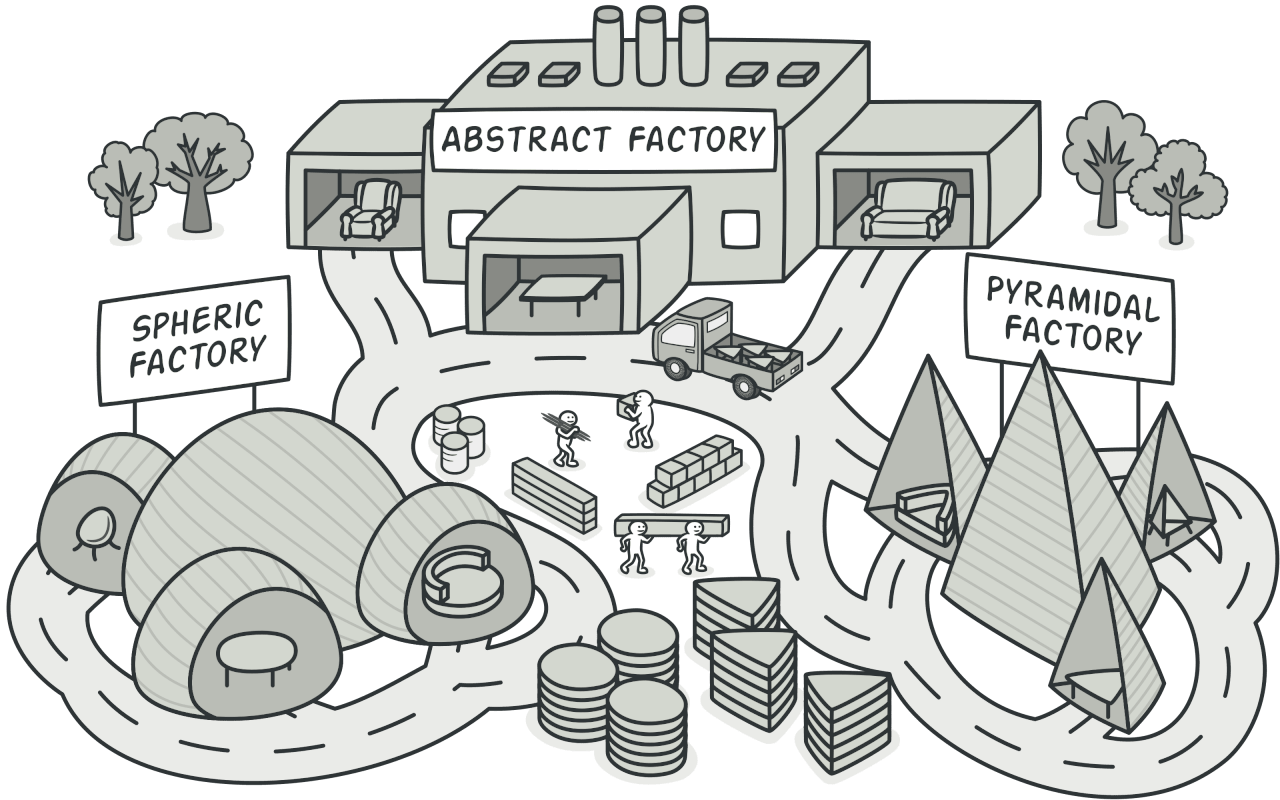
Deep Dive into Creational Patterns: The Abstract Factory.
Hey software designers! Today, we’re exploring the Abstract Factory pattern in depth. This pattern is a cornerstone in the world of design patterns, providing a robust way to create families of related or dependent objects without specifying their concrete classes. Let’s uncover its intricacies, benefits, and real-world applications with detailed examples.
What is the Abstract Factory Pattern?
The Abstract Factory pattern is a creational design pattern that provides an interface for creating families of related or dependent objects without specifying their concrete classes. It encapsulates a group of individual factories with a common goal. In essence, the pattern defines a high-level interface to create objects, but delegates the actual creation to specific subclasses.
Real-World Scenario
Imagine you’re tasked with developing a cross-platform GUI application. You need to support multiple operating systems (Windows, macOS, Linux), each having its own set of UI components like buttons and checkboxes. Without a systematic approach, you might end up with a lot of platform-specific code scattered throughout your application, making it hard to maintain and extend.
The Problem
When building a cross-platform GUI toolkit, different platforms have different UI controls. For instance, a button in Windows looks different from a button in macOS. Hardcoding the creation of these UI elements for each platform can lead to a mess of if-else statements or switch cases, making your codebase fragile and hard to maintain.
Without Abstract Factory
class Application
def initialize(os)
@os = os
end
def create_ui
if @os == 'Windows'
button = WindowsButton.new
checkbox = WindowsCheckbox.new
elsif @os == 'Mac'
button = MacButton.new
checkbox = MacCheckbox.new
else
raise 'Unknown operating system'
end
button.paint
checkbox.paint
end
end
class WindowsButton
def paint
puts 'Render a button in Windows style'
end
end
class WindowsCheckbox
def paint
puts 'Render a checkbox in Windows style'
end
end
class MacButton
def paint
puts 'Render a button in macOS style'
end
end
class MacCheckbox
def paint
puts 'Render a checkbox in macOS style'
end
end
app = Application.new('Windows')
app.create_ui
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new platforms.
The Solution: Abstract Factory Pattern
Using the Abstract Factory pattern, we can encapsulate the platform-specific creation logic into separate factory classes. This way, the client code remains agnostic to the actual product classes.
With Abstract Factory
Step 1: Define Abstract Products
# Abstract product interfaces
class Button
def paint
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
class Checkbox
def paint
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Products
# Concrete product implementations for Windows
class WindowsButton < Button
def paint
puts 'Render a button in Windows style'
end
end
class WindowsCheckbox < Checkbox
def paint
puts 'Render a checkbox in Windows style'
end
end
# Concrete product implementations for macOS
class MacButton < Button
def paint
puts 'Render a button in macOS style'
end
end
class MacCheckbox < Checkbox
def paint
puts 'Render a checkbox in macOS style'
end
end
Step 3: Define Abstract Factory
# Abstract factory interface
class GUIFactory
def create_button
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def create_checkbox
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 4: Create Concrete Factories
# Concrete factory implementations for Windows
class WindowsFactory < GUIFactory
def create_button
WindowsButton.new
end
def create_checkbox
WindowsCheckbox.new
end
end
# Concrete factory implementations for macOS
class MacFactory < GUIFactory
def create_button
MacButton.new
end
def create_checkbox
MacCheckbox.new
end
end
Step 5: Implement Client Code
# Client code that uses the factory
class Application
def initialize(factory)
@factory = factory
@button = @factory.create_button
@checkbox = @factory.create_checkbox
end
def paint
@button.paint
@checkbox.paint
end
end
# Example usage
def configure_application(os)
factory = case os
when 'Windows' then WindowsFactory.new
when 'Mac' then MacFactory.new
else raise 'Unknown operating system'
end
app = Application.new(factory)
app.paint
end
configure_application('Windows')
Real-World Benefits
Scenario: Switching UI Themes
Imagine you need to switch from a light theme to a dark theme in your application. Using the Abstract Factory pattern, you can easily create a new set of products (buttons, checkboxes, etc.) for the dark theme and switch factories without changing the client code.
Without Abstract Factory:
class Application
def paint
if theme == 'light'
button = LightButton.new
checkbox = LightCheckbox.new
elsif theme == 'dark'
button = DarkButton.new
checkbox = DarkCheckbox.new
end
button.paint
checkbox.paint
end
end
Drawbacks: Code is tightly coupled, difficult to maintain, and modify.
With Abstract Factory:
def configure_application(theme)
factory = case theme
when 'light' then LightThemeFactory.new
when 'dark' then DarkThemeFactory.new
else raise 'Unknown theme'
end
app = Application.new(factory)
app.paint
end
Benefits: Clean, maintainable code with high flexibility.
Conclusion
The Abstract Factory pattern is a powerful tool in the software designer’s toolkit. It helps in creating families of related objects without being tied to their concrete implementations. This pattern promotes flexibility, consistency, and decoupling, making your code easier to manage and extend. Incorporate the Abstract Factory pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!