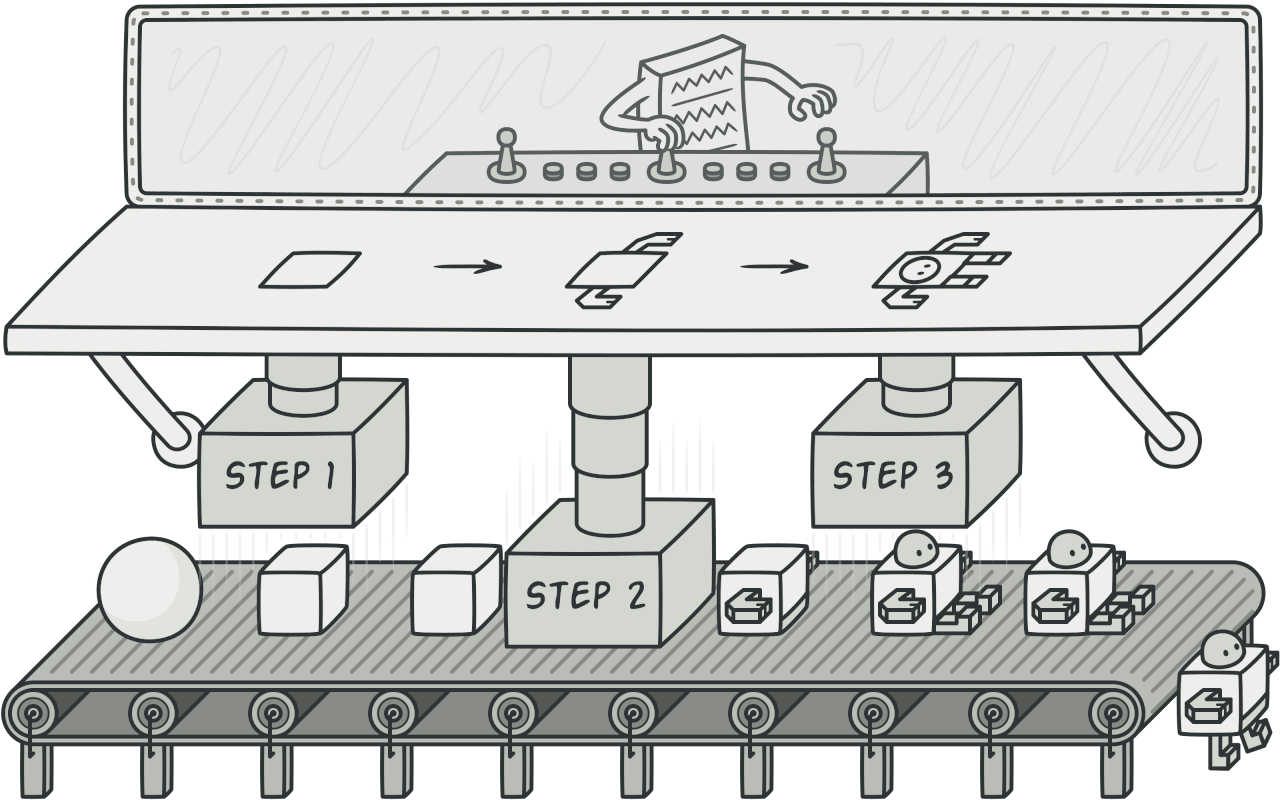
Deep Dive into Creational Patterns: The Builder Pattern.
Hey software designers! Today, we’re diving into the Builder pattern. This pattern is essential for constructing complex objects step by step, providing a flexible solution to object creation. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Builder Pattern?
The Builder pattern is a creational design pattern that allows you to construct complex objects step by step. Unlike other creational patterns, the Builder pattern doesn’t require products to have a common interface. It separates the construction of a complex object from its representation, enabling the same construction process to create different representations.
Real-World Scenario
Imagine you’re building a customizable burger at a fast-food restaurant. A customer can choose from a variety of ingredients (buns, patties, vegetables, sauces). Without a systematic approach, you might end up with a disorganized process, leading to incorrect orders and unhappy customers.
The Problem
When constructing complex objects like customizable burgers, managing the creation process can become chaotic. Hardcoding the creation logic can result in a monolithic and inflexible codebase.
Without Builder Pattern
class Burger
attr_accessor :bun, :patty, :vegetables, :sauce
def initialize(bun, patty, vegetables, sauce)
@bun = bun
@patty = patty
@vegetables = vegetables
@sauce = sauce
end
end
burger = Burger.new('Sesame', 'Beef', ['Lettuce', 'Tomato'], 'Mayo')
Drawbacks: The constructor becomes unwieldy with many parameters, making it hard to read and maintain.
The Solution: Builder Pattern
Using the Builder pattern, we can construct complex objects step by step, allowing for greater flexibility and readability.
With Builder Pattern
Step 1: Define the Product
class Burger
attr_accessor :bun, :patty, :vegetables, :sauce
def initialize
@vegetables = []
end
def describe
"Burger with #{@bun} bun, #{@patty} patty, #{vegetables.join(', ')} vegetables, and #{@sauce} sauce."
end
end
Step 2: Create the Builder Interface
class BurgerBuilder
def add_bun(bun)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def add_patty(patty)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def add_vegetables(vegetables)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def add_sauce(sauce)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def build
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 3: Implement Concrete Builders
class ConcreteBurgerBuilder < BurgerBuilder
def initialize
@burger = Burger.new
end
def add_bun(bun)
@burger.bun = bun
self
end
def add_patty(patty)
@burger.patty = patty
self
end
def add_vegetables(vegetables)
@burger.vegetables.concat(vegetables)
self
end
def add_sauce(sauce)
@burger.sauce = sauce
self
end
def build
@burger
end
end
Step 4: Create the Director
class BurgerDirector
def initialize(builder)
@builder = builder
end
def construct
@builder.add_bun('Sesame')
.add_patty('Beef')
.add_vegetables(['Lettuce', 'Tomato'])
.add_sauce('Mayo')
.build
end
end
Step 5: Implement Client Code
builder = ConcreteBurgerBuilder.new
director = BurgerDirector.new(builder)
burger = director.construct
puts burger.describe
Real-World Benefits
Scenario: Creating Customizable Orders
Imagine you need to offer various types of burgers (vegan, chicken, beef) with different combinations of ingredients. Using the Builder pattern, you can easily construct different types of burgers without altering the client code.
Without Builder Pattern:
class Burger
def initialize(type)
case type
when 'vegan'
@bun = 'Whole Wheat'
@patty = 'Black Bean'
@vegetables = ['Lettuce', 'Tomato']
@sauce = 'Hummus'
when 'chicken'
@bun = 'Sesame'
@patty = 'Chicken'
@vegetables = ['Lettuce', 'Pickles']
@sauce = 'Mayo'
else
@bun = 'Sesame'
@patty = 'Beef'
@vegetables = ['Lettuce', 'Tomato']
@sauce = 'Mayo'
end
end
end
burger = Burger.new('vegan')
Drawbacks: The constructor becomes cluttered with conditional logic, making it difficult to extend and maintain.
With Builder Pattern:
builder = ConcreteBurgerBuilder.new
director = BurgerDirector.new(builder)
vegan_burger = director.construct('vegan')
puts vegan_burger.describe
Benefits: Clean, maintainable code with high flexibility and readability.
Conclusion
The Builder pattern is a powerful tool for constructing complex objects step by step. It promotes flexibility, readability, and maintainability in your code. By separating the construction of a complex object from its representation, the Builder pattern allows for greater control over the object creation process. Incorporate the Builder pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!