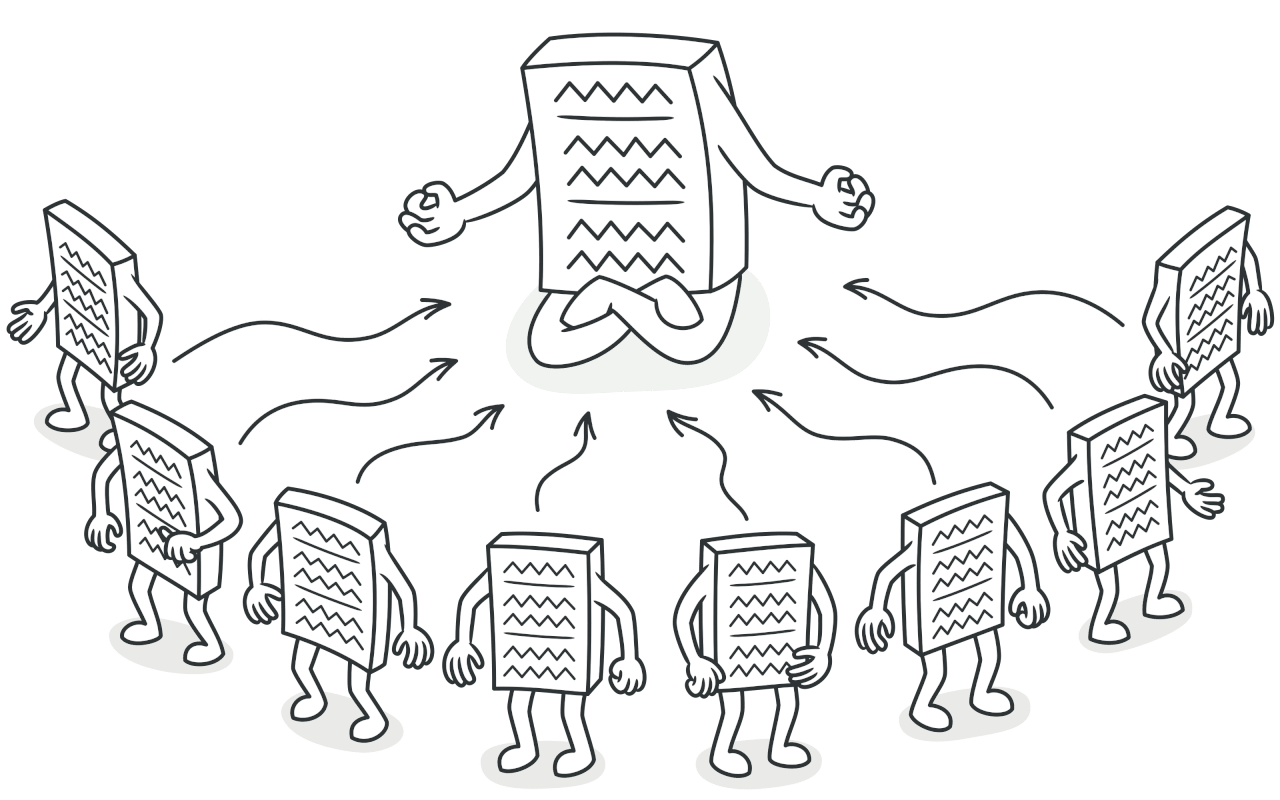
Deep Dive into Creational Patterns - The Singleton Pattern.
Hey software designers! Today, we’re exploring the Singleton pattern. This pattern ensures that a class has only one instance and provides a global point of access to it. Let’s dive into its workings, benefits, and real-world applications with detailed examples.
What is the Singleton Pattern?
The Singleton pattern is a creational design pattern that restricts the instantiation of a class to a single instance. This is useful when exactly one object is needed to coordinate actions across the system. The Singleton pattern ensures that a class has only one instance and provides a global point of access to it.
Real-World Scenario
Imagine you’re developing a logging system for an application. The logging system should only have one instance to ensure that all parts of the application log messages to the same place. Without a systematic approach, you might end up with multiple loggers, leading to inconsistent and hard-to-track logs.
The Problem
When a class must have only one instance, creating multiple instances can lead to problems such as inconsistent state, increased memory usage, and difficulty in managing shared resources. Hardcoding a single instance throughout the application can lead to tight coupling and inflexibility.
Without Singleton Pattern
class Logger
def log(message)
puts "Log: #{message}"
end
end
logger1 = Logger.new
logger2 = Logger.new
logger1.log('First message')
logger2.log('Second message')
Drawbacks: Multiple instances of the Logger class can lead to inconsistent logging and difficulty in managing the logging process.
The Solution: Singleton Pattern
Using the Singleton pattern, we can ensure that only one instance of the Logger class exists, providing a consistent and manageable logging process.
With Singleton Pattern
Step 1: Define the Singleton Class
class Logger
@instance = Logger.new
private_class_method :new
def self.instance
@instance
end
def log(message)
puts "Log: #{message}"
end
end
Step 2: Implement Client Code
logger1 = Logger.instance
logger2 = Logger.instance
logger1.log('First message')
logger2.log('Second message')
Benefits: Ensures that only one instance of the Logger class exists, providing consistent and manageable logging.
Real-World Benefits
Scenario: Managing Configuration Settings
Imagine you need to manage configuration settings for your application. Using the Singleton pattern, you can ensure that only one instance of the configuration manager exists, providing a consistent and manageable way to access and update settings.
Without Singleton Pattern:
class ConfigManager
def initialize
@settings = {}
end
def set(key, value)
@settings[key] = value
end
def get(key)
@settings[key]
end
end
config1 = ConfigManager.new
config2 = ConfigManager.new
config1.set('theme', 'dark')
puts config2.get('theme') # nil
Drawbacks: Multiple instances of the ConfigManager class can lead to inconsistent settings and difficulty in managing the configuration process.
With Singleton Pattern:
class ConfigManager
@instance = ConfigManager.new
private_class_method :new
def self.instance
@instance
end
def initialize
@settings = {}
end
def set(key, value)
@settings[key] = value
end
def get(key)
@settings[key]
end
end
config1 = ConfigManager.instance
config2 = ConfigManager.instance
config1.set('theme', 'dark')
puts config2.get('theme') # dark
Benefits: Ensures that only one instance of the ConfigManager class exists, providing consistent and manageable configuration settings.
Conclusion
The Singleton pattern is a powerful tool for ensuring that a class has only one instance and providing a global point of access to it. It promotes consistency, efficiency, and manageability in your code. By using the Singleton pattern, you can ensure that shared resources are used effectively and that your application behaves predictably. Incorporate the Singleton pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!