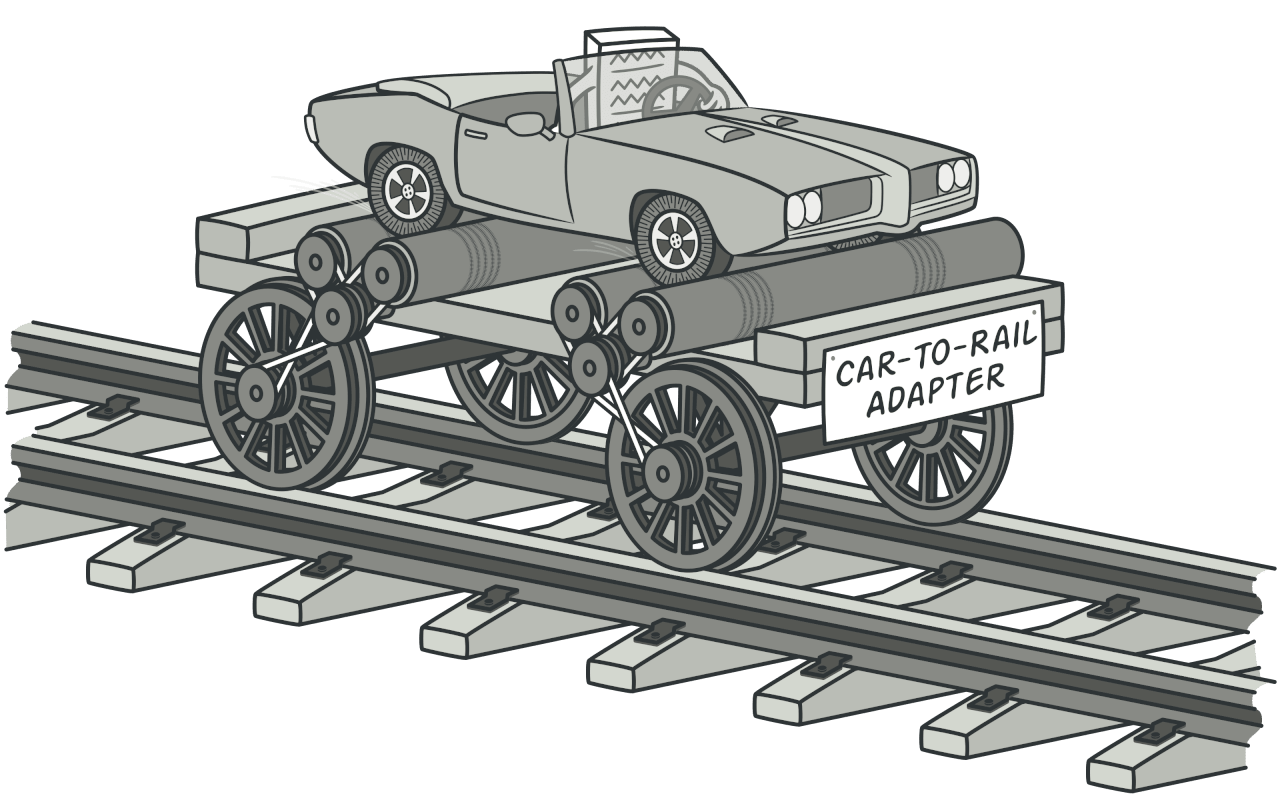
Deep Dive into Structural Patterns - The Adapter Pattern.
Hey software designers! Today, we’re diving into the Adapter pattern. This pattern allows incompatible interfaces to work together, providing a flexible solution to interface mismatches. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Adapter Pattern?
The Adapter pattern is a structural design pattern that allows objects with incompatible interfaces to work together. It acts as a bridge between two incompatible interfaces by wrapping an existing class with a new interface.
Real-World Scenario
Imagine you’re developing a payment processing system that needs to integrate with multiple third-party payment gateways. Each payment gateway has a different API, making it challenging to integrate them directly into your system.
The Problem
When integrating multiple third-party services with different interfaces, directly using these services can lead to a tangled and hard-to-maintain codebase. Hardcoding the integration logic can result in tightly coupled and inflexible code.
Without Adapter Pattern
class PayPalPayment
def send_payment(amount)
puts "Sending payment of #{amount} via PayPal"
end
end
class StripePayment
def process_payment(amount)
puts "Processing payment of #{amount} via Stripe"
end
end
class PaymentProcessor
def process(amount, gateway)
case gateway
when 'PayPal'
PayPalPayment.new.send_payment(amount)
when 'Stripe'
StripePayment.new.process_payment(amount)
else
raise 'Unknown payment gateway'
end
end
end
processor = PaymentProcessor.new
processor.process(100, 'PayPal')
processor.process(200, 'Stripe')
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new payment gateways.
The Solution: Adapter Pattern
Using the Adapter pattern, we can create a unified interface for different payment gateways, making the code more flexible and maintainable.
With Adapter Pattern
Step 1: Define the Target Interface
class PaymentGateway
def pay(amount)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Adapters for Each Payment Gateway
class PayPalAdapter < PaymentGateway
def initialize(paypal_payment)
@paypal_payment = paypal_payment
end
def pay(amount)
@paypal_payment.send_payment(amount)
end
end
class StripeAdapter < PaymentGateway
def initialize(stripe_payment)
@stripe_payment = stripe_payment
end
def pay(amount)
@stripe_payment.process_payment(amount)
end
end
Step 3: Implement Client Code
class PaymentProcessor
def initialize(gateway)
@gateway = gateway
end
def process(amount)
@gateway.pay(amount)
end
end
paypal = PayPalAdapter.new(PayPalPayment.new)
stripe = StripeAdapter.new(StripePayment.new)
processor = PaymentProcessor.new(paypal)
processor.process(100)
processor = PaymentProcessor.new(stripe)
processor.process(200)
Benefits: Promotes loose coupling and makes the code more flexible and maintainable.
Real-World Benefits
Scenario: Adding New Payment Gateways
Imagine you need to add a new payment gateway (e.g., Square). Using the Adapter pattern, you can easily introduce a new adapter for the Square payment gateway without modifying the existing code.
Without Adapter Pattern:
class PaymentProcessor
def process(amount, gateway)
case gateway
when 'PayPal'
PayPalPayment.new.send_payment(amount)
when 'Stripe'
StripePayment.new.process_payment(amount)
when 'Square'
SquarePayment.new.make_payment(amount)
else
raise 'Unknown payment gateway'
end
end
end
class SquarePayment
def make_payment(amount)
puts "Making payment of #{amount} via Square"
end
end
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Adapter Pattern:
class SquareAdapter < PaymentGateway
def initialize(square_payment)
@square_payment = square_payment
end
def pay(amount)
@square_payment.make_payment(amount)
end
end
square = SquareAdapter.new(SquarePayment.new)
processor = PaymentProcessor.new(square)
processor.process(300)
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Adapter pattern is a powerful tool for allowing incompatible interfaces to work together. It promotes flexibility, maintainability, and loose coupling in your code. By using the Adapter pattern, you can easily integrate third-party services and components with different interfaces into your system. Incorporate the Adapter pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!