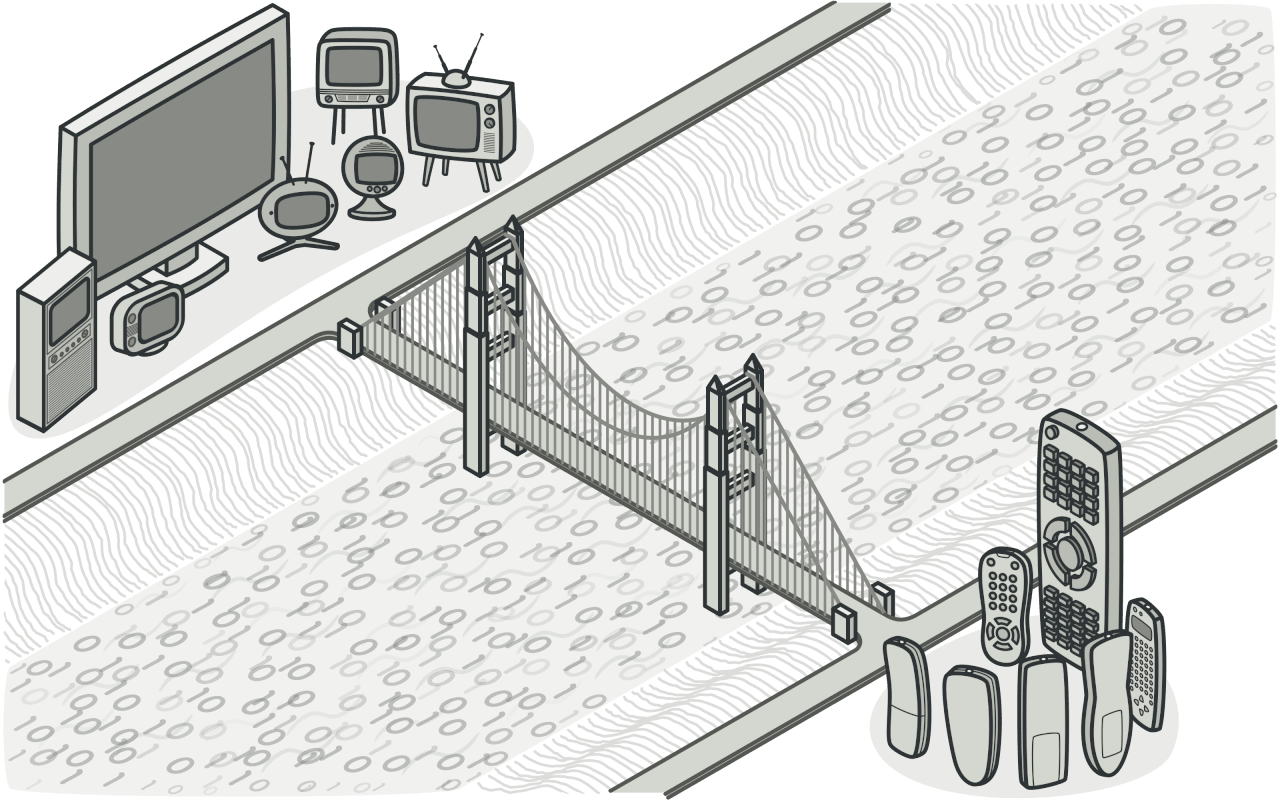
Deep Dive into Structural Patterns - The Bridge Pattern.
Hey software designers! Today, we’re diving into the Bridge pattern. This pattern allows you to separate an object’s abstraction from its implementation, promoting flexibility and scalability. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Bridge Pattern?
The Bridge pattern is a structural design pattern that decouples an abstraction from its implementation so that the two can vary independently. It achieves this by creating two separate class hierarchies: one for the abstraction and one for the implementation.
Real-World Scenario
Imagine you’re developing a remote control system for various electronic devices (TVs, radios, lights). Each device has different functionalities and interfaces. Directly integrating these devices with the remote control can lead to a tightly coupled and inflexible system.
The Problem
When an application needs to support multiple abstractions and implementations that should vary independently, directly integrating these abstractions and implementations can lead to a complex and tightly coupled system. This approach makes it difficult to introduce new abstractions or implementations without modifying existing code.
Without Bridge Pattern
class RemoteControl
def initialize(device)
@device = device
end
def turn_on
if @device == 'TV'
puts 'Turning on the TV'
elsif @device == 'Radio'
puts 'Turning on the Radio'
else
raise 'Unknown device'
end
end
end
remote = RemoteControl.new('TV')
remote.turn_on
remote = RemoteControl.new('Radio')
remote.turn_on
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new devices.
The Solution: Bridge Pattern
Using the Bridge pattern, we can decouple the abstraction (remote control) from its implementation (devices), promoting flexibility and scalability.
With Bridge Pattern
Step 1: Define the Implementor Interface
class Device
def turn_on
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Implementors
class TV < Device
def turn_on
puts 'Turning on the TV'
end
end
class Radio < Device
def turn_on
puts 'Turning on the Radio'
end
end
Step 3: Define the Abstraction
class RemoteControl
def initialize(device)
@device = device
end
def turn_on
@device.turn_on
end
end
Step 4: Implement Client Code
tv = TV.new
radio = Radio.new
remote = RemoteControl.new(tv)
remote.turn_on
remote = RemoteControl.new(radio)
remote.turn_on
Benefits: Decouples the abstraction from its implementation, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Devices
Imagine you need to add a new device (e.g., Light). Using the Bridge pattern, you can easily introduce a new implementor for the Light device without modifying the existing code.
Without Bridge Pattern:
class RemoteControl
def initialize(device)
@device = device
end
def turn_on
case @device
when 'TV'
puts 'Turning on the TV'
when 'Radio'
puts 'Turning on the Radio'
when 'Light'
puts 'Turning on the Light'
else
raise 'Unknown device'
end
end
end
remote = RemoteControl.new('Light')
remote.turn_on
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Bridge Pattern:
class Light < Device
def turn_on
puts 'Turning on the Light'
end
end
light = Light.new
remote = RemoteControl.new(light)
remote.turn_on
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Bridge pattern is a powerful tool for decoupling abstractions from implementations. It promotes flexibility, scalability, and maintainability in your code. By using the Bridge pattern, you can easily introduce new abstractions and implementations without modifying existing code. Incorporate the Bridge pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!