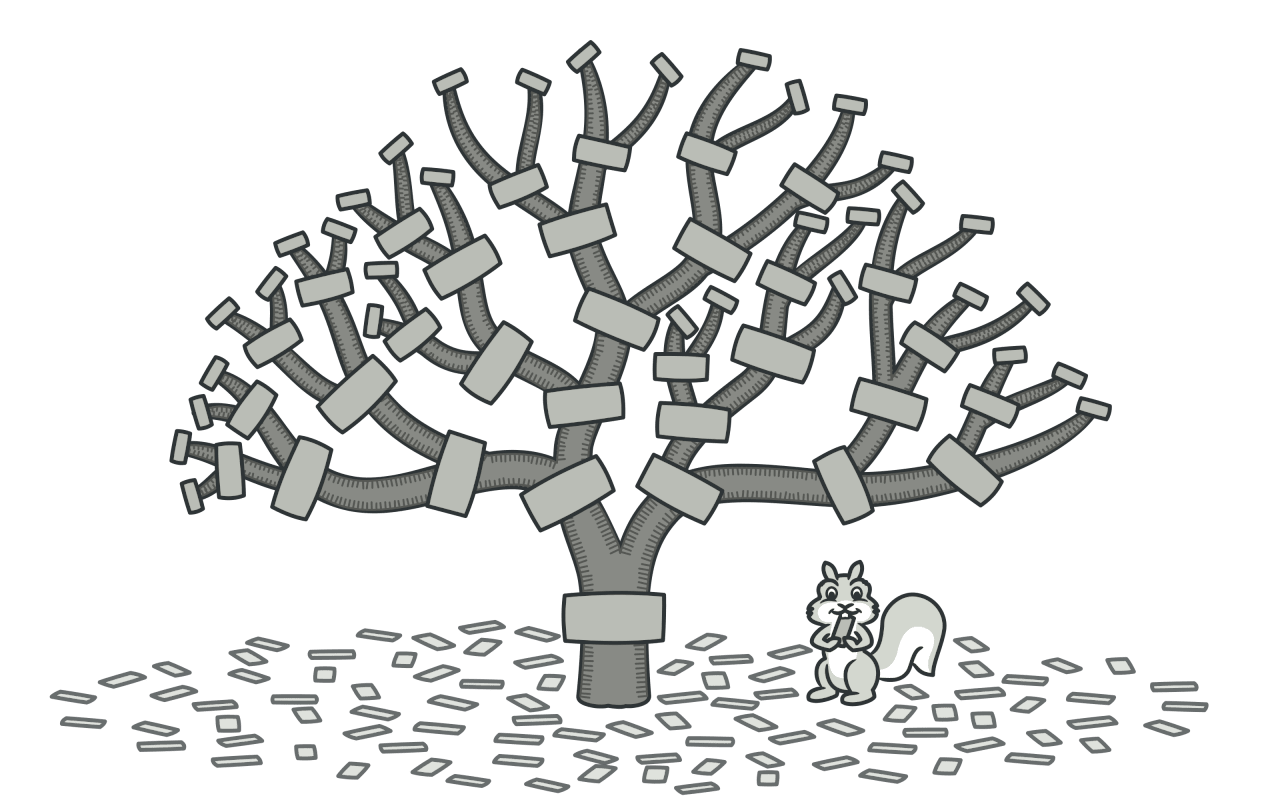
Deep Dive into Structural Patterns - The Composite Pattern.
Hey software designers! Today, we’re diving into the Composite pattern. This pattern allows you to compose objects into tree structures to represent part-whole hierarchies. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Composite Pattern?
The Composite pattern is a structural design pattern that allows you to compose objects into tree structures to represent part-whole hierarchies. It lets clients treat individual objects and compositions of objects uniformly.
Real-World Scenario
Imagine you’re developing a graphics editor that allows users to draw shapes like circles, rectangles, and groups of shapes. Each shape can be an individual object or a composition of multiple shapes. Managing these shapes in a consistent and flexible way can be challenging.
The Problem
When managing individual objects and compositions of objects, treating them differently can lead to a complex and hard-to-maintain codebase. Hardcoding the logic to handle different types of objects can result in tightly coupled and inflexible code.
Without Composite Pattern
class Circle
def draw
puts 'Drawing a circle'
end
end
class Rectangle
def draw
puts 'Drawing a rectangle'
end
end
class Group
def initialize
@shapes = []
end
def add(shape)
@shapes << shape
end
def draw
@shapes.each(&:draw)
end
end
circle = Circle.new
rectangle = Rectangle.new
group = Group.new
group.add(circle)
group.add(rectangle)
group.draw
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new shapes.
The Solution: Composite Pattern
Using the Composite pattern, we can treat individual objects and compositions of objects uniformly, promoting flexibility and scalability.
With Composite Pattern
Step 1: Define the Component Interface
class Shape
def draw
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Leaf Components
class Circle < Shape
def draw
puts 'Drawing a circle'
end
end
class Rectangle < Shape
def draw
puts 'Drawing a rectangle'
end
end
Step 3: Create Composite Components
class Group < Shape
def initialize
@shapes = []
end
def add(shape)
@shapes << shape
end
def draw
@shapes.each(&:draw)
end
end
Step 4: Implement Client Code
circle = Circle.new
rectangle = Rectangle.new
group = Group.new
group.add(circle)
group.add(rectangle)
group.draw
Benefits: Treats individual objects and compositions of objects uniformly, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Shapes
Imagine you need to add a new shape (e.g., Triangle). Using the Composite pattern, you can easily introduce a new shape without modifying the existing code.
Without Composite Pattern:
class Triangle
def draw
puts 'Drawing a triangle'
end
end
group = Group.new
triangle = Triangle.new
group.add(triangle)
group.draw
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Composite Pattern:
class Triangle < Shape
def draw
puts 'Drawing a triangle'
end
end
triangle = Triangle.new
group.add(triangle)
group.draw
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Composite pattern is a powerful tool for composing objects into tree structures to represent part-whole hierarchies. It promotes flexibility, scalability, and maintainability in your code. By using the Composite pattern, you can easily manage individual objects and compositions of objects uniformly. Incorporate the Composite pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!