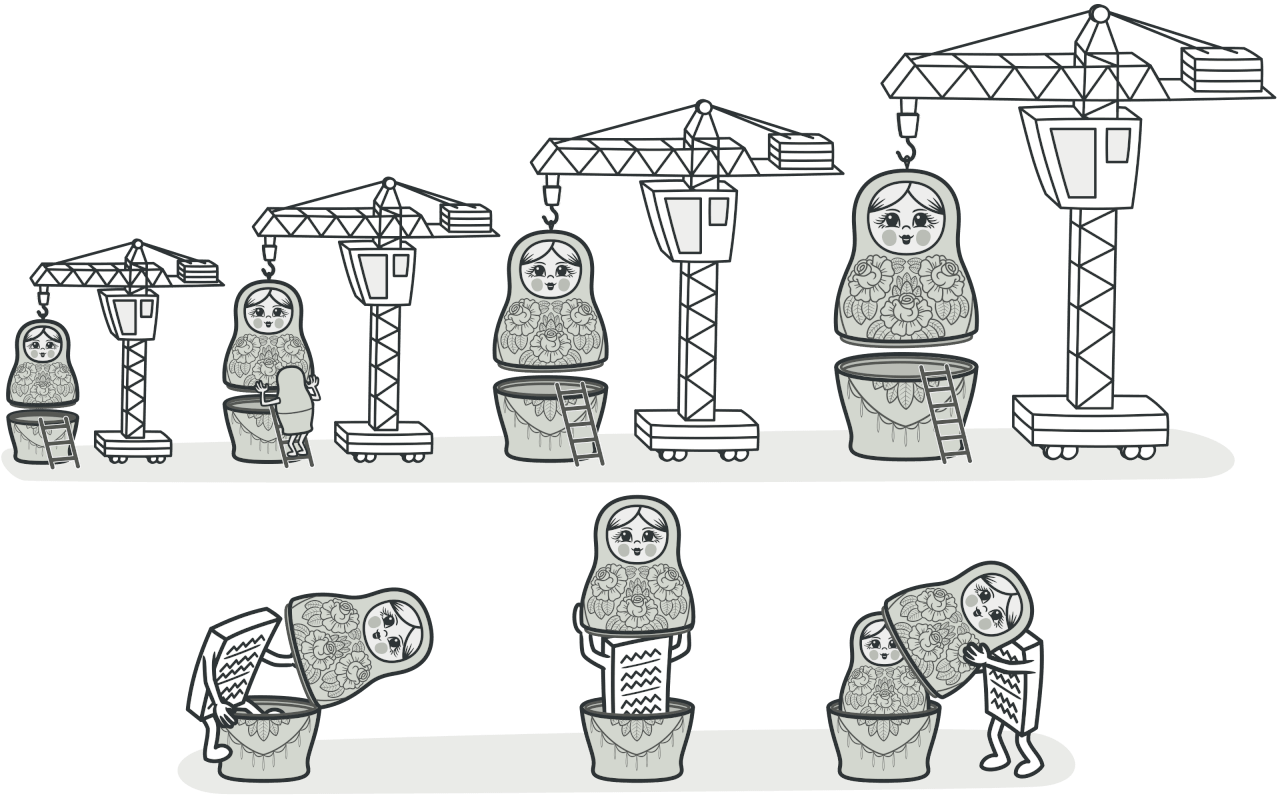
Deep Dive into Structural Patterns - The Decorator Pattern.
Hey software designers! Today, we’re diving into the Decorator pattern. This pattern allows you to add new functionality to objects dynamically and transparently. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Decorator Pattern?
The Decorator pattern is a structural design pattern that allows you to dynamically add behavior to objects without altering their code. It provides a flexible alternative to subclassing for extending functionality.
Real-World Scenario
Imagine you’re developing a text editor that allows users to add various formatting options to text (e.g., bold, italic, underline). Each formatting option can be applied independently or in combination with others. Managing these formatting options can be challenging.
The Problem
When extending the functionality of objects, subclassing can lead to a rigid and inflexible class hierarchy. Hardcoding the logic to handle different combinations of behavior can result in tightly coupled and hard-to-maintain code.
Without Decorator Pattern
class Text
def initialize(content)
@content = content
end
def display
@content
end
end
class BoldText < Text
def display
"<b>#{super}</b>"
end
end
class ItalicText < Text
def display
"<i>#{super}</i>"
end
end
bold_text = BoldText.new("Hello")
puts bold_text.display
italic_text = ItalicText.new("Hello")
puts italic_text.display
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new formatting options.
The Solution: Decorator Pattern
Using the Decorator pattern, we can dynamically add behavior to objects without altering their code, promoting flexibility and scalability.
With Decorator Pattern
Step 1: Define the Component Interface
class Text
def display
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Components
class PlainText < Text
def initialize(content)
@content = content
end
def display
@content
end
end
Step 3: Create Decorators
class TextDecorator < Text
def initialize(text)
@text = text
end
def display
@text.display
end
end
class BoldDecorator < TextDecorator
def display
"<b>#{super}</b>"
end
end
class ItalicDecorator < TextDecorator
def display
"<i>#{super}</i>"
end
end
Step 4: Implement Client Code
plain_text = PlainText.new("Hello")
bold_text = BoldDecorator.new(plain_text)
puts bold_text.display
italic_text = ItalicDecorator.new(plain_text)
puts italic_text.display
bold_italic_text = ItalicDecorator.new(bold_text)
puts bold_italic_text.display
Benefits: Dynamically adds behavior to objects without altering their code, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Formatting Options
Imagine you need to add a new formatting option (e.g., Underline). Using the Decorator pattern, you can easily introduce a new decorator for the Underline formatting without modifying the existing code.
Without Decorator Pattern:
class UnderlineText < Text
def display
"<u>#{super}</u>"
end
end
underline_text = UnderlineText.new("Hello")
puts underline_text.display
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Decorator Pattern:
class UnderlineDecorator < TextDecorator
def display
"<u>#{super}</u>"
end
end
underline_text = UnderlineDecorator.new(plain_text)
puts underline_text.display
bold_underline_text = UnderlineDecorator.new(bold_text)
puts bold_underline_text.display
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Decorator pattern is a powerful tool for dynamically adding behavior to objects without altering their code. It promotes flexibility, scalability, and maintainability in your code. By using the Decorator pattern, you can easily extend the functionality of objects without creating a rigid and inflexible class hierarchy. Incorporate the Decorator pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!