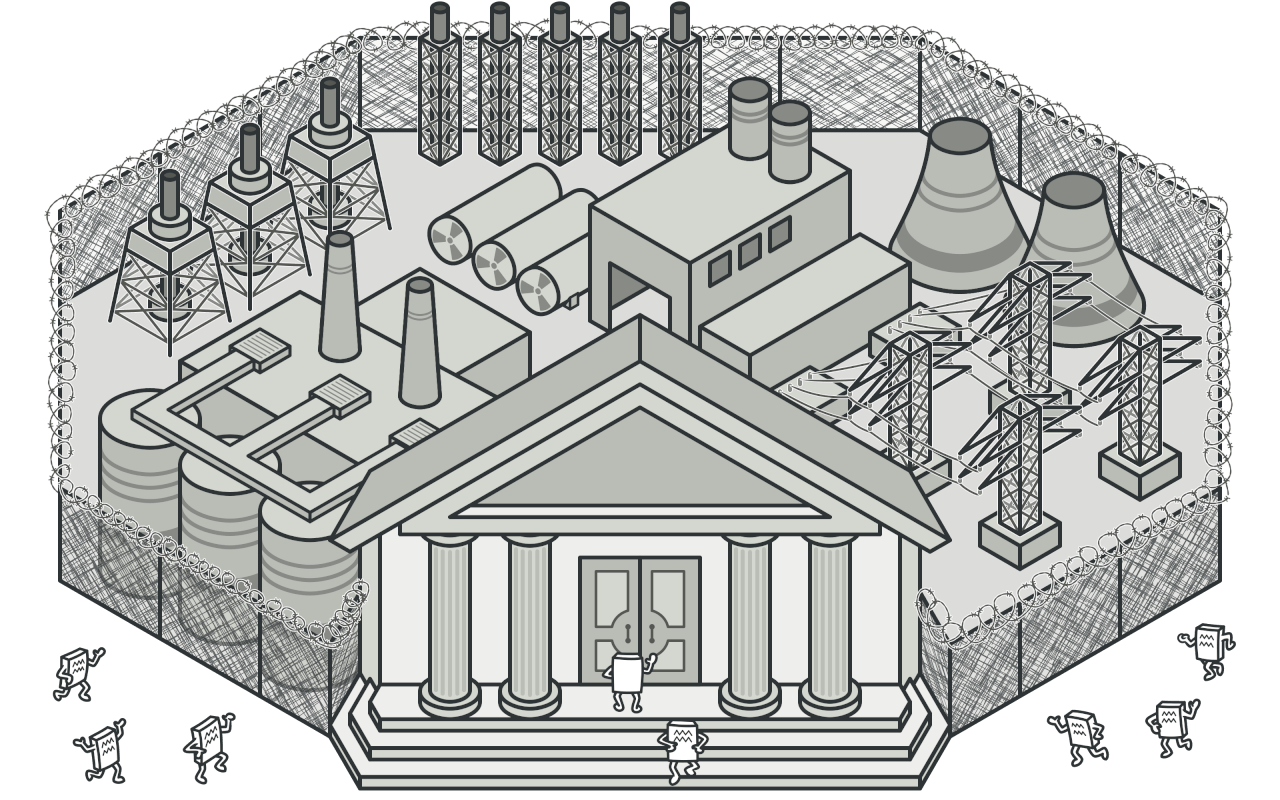
Deep Dive into Structural Patterns - The Facade Pattern.
Hey software designers! Today, we’re diving into the Facade pattern. This pattern provides a simplified interface to a complex subsystem, making it easier to use. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Facade Pattern?
The Facade pattern is a structural design pattern that provides a simplified interface to a complex subsystem. It defines a higher-level interface that makes the subsystem easier to use by hiding the complexity behind a simpler API.
Real-World Scenario
Imagine you’re developing a home automation system that controls various devices like lights, thermostats, and security cameras. Each device has its own interface and functionality, making it difficult for users to control them through a single interface.
The Problem
When dealing with complex subsystems, directly interacting with their interfaces can lead to a complicated and hard-to-maintain codebase. Hardcoding the logic to handle different parts of the subsystem can result in tightly coupled and inflexible code.
Without Facade Pattern
class Light
def turn_on
puts 'Turning on the light'
end
def turn_off
puts 'Turning off the light'
end
end
class Thermostat
def set_temperature(temperature)
puts "Setting temperature to #{temperature} degrees"
end
end
class SecurityCamera
def activate
puts 'Activating security camera'
end
def deactivate
puts 'Deactivating security camera'
end
end
light = Light.new
thermostat = Thermostat.new
camera = SecurityCamera.new
light.turn_on
thermostat.set_temperature(22)
camera.activate
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new devices.
The Solution: Facade Pattern
Using the Facade pattern, we can provide a simplified interface to the complex subsystem, promoting flexibility and scalability.
With Facade Pattern
Step 1: Define the Subsystem Interfaces
class Light
def turn_on
puts 'Turning on the light'
end
def turn_off
puts 'Turning off the light'
end
end
class Thermostat
def set_temperature(temperature)
puts "Setting temperature to #{temperature} degrees"
end
end
class SecurityCamera
def activate
puts 'Activating security camera'
end
def deactivate
puts 'Deactivating security camera'
end
end
Step 2: Create the Facade
class HomeAutomationFacade
def initialize
@light = Light.new
@thermostat = Thermostat.new
@camera = SecurityCamera.new
end
def leave_home
@light.turn_off
@thermostat.set_temperature(18)
@camera.activate
end
def arrive_home
@light.turn_on
@thermostat.set_temperature(22)
@camera.deactivate
end
end
Step 3: Implement Client Code
home = HomeAutomationFacade.new
home.leave_home
home.arrive_home
Benefits: Provides a simplified interface to a complex subsystem, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Devices
Imagine you need to add a new device (e.g., Smart Lock). Using the Facade pattern, you can easily extend the home automation system without modifying the existing code.
Without Facade Pattern:
class SmartLock
def lock
puts 'Locking the smart lock'
end
def unlock
puts 'Unlocking the smart lock'
end
end
lock = SmartLock.new
lock.lock
lock.unlock
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Facade Pattern:
class HomeAutomationFacade
def initialize
@light = Light.new
@thermostat = Thermostat.new
@camera = SecurityCamera.new
@lock = SmartLock.new
end
def leave_home
@light.turn_off
@thermostat.set_temperature(18)
@camera.activate
@lock.lock
end
def arrive_home
@light.turn_on
@thermostat.set_temperature(22)
@camera.deactivate
@lock.unlock
end
end
home = HomeAutomationFacade.new
home.leave_home
home.arrive_home
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Facade pattern is a powerful tool for providing a simplified interface to a complex subsystem. It promotes flexibility, scalability, and maintainability in your code. By using the Facade pattern, you can easily extend and manage complex subsystems through a unified and simplified interface. Incorporate the Facade pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!