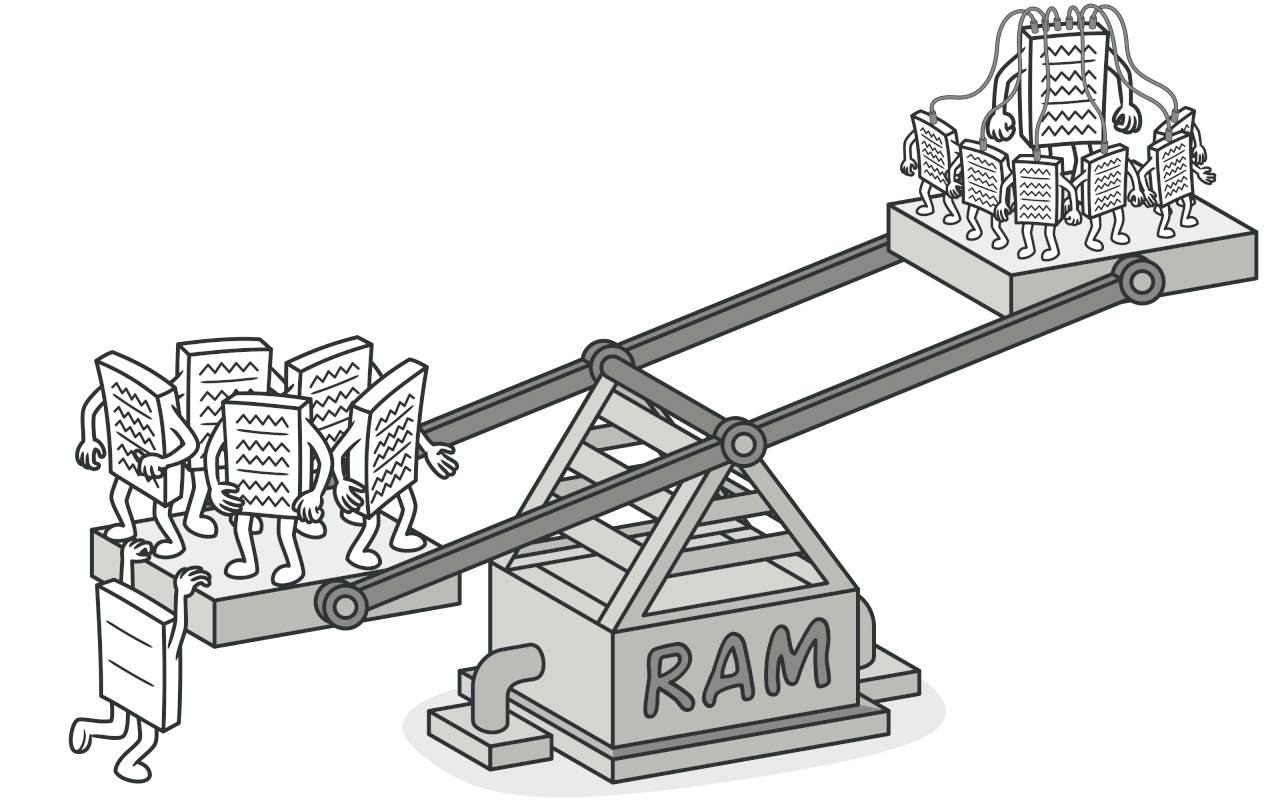
Deep Dive into Structural Patterns - The Flyweight Pattern.
Hey software designers! Today, we’re diving into the Flyweight pattern. This pattern helps you efficiently support a large number of fine-grained objects by sharing as much data as possible. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Flyweight Pattern?
The Flyweight pattern is a structural design pattern that allows you to minimize memory usage by sharing as much data as possible with similar objects. It is particularly useful when you need to create a large number of similar objects that would otherwise consume a significant amount of memory.
Real-World Scenario
Imagine you’re developing a graphical application that needs to render a large number of characters on the screen. Each character has its own properties (e.g., font, size, color), and creating an individual object for each character can lead to high memory consumption.
The Problem
When creating a large number of fine-grained objects, each with its own state, memory consumption can become a significant issue. Hardcoding the logic to handle these objects can result in a tightly coupled and inefficient codebase.
Without Flyweight Pattern
class Character
def initialize(char, font, size, color)
@char = char
@font = font
@size = size
@color = color
end
def display
puts "Displaying #{@char} in #{@font} font, size #{@size}, color #{@color}"
end
end
characters = []
('a'..'z').each do |char|
characters << Character.new(char, 'Arial', 12, 'black')
end
characters.each(&:display)
Drawbacks: Each character object consumes memory for storing its properties, leading to high memory consumption.
The Solution: Flyweight Pattern
Using the Flyweight pattern, we can share the common properties of similar objects, reducing memory consumption and improving efficiency.
With Flyweight Pattern
Step 1: Define the Flyweight
class CharacterFlyweight
def initialize(char)
@char = char
end
def display(font, size, color)
puts "Displaying #{@char} in #{font} font, size #{size}, color #{color}"
end
end
Step 2: Create the Flyweight Factory
class FlyweightFactory
def initialize
@flyweights = {}
end
def get_flyweight(char)
@flyweights[char] ||= CharacterFlyweight.new(char)
end
end
Step 3: Implement Client Code
factory = FlyweightFactory.new
characters = []
('a'..'z').each do |char|
characters << factory.get_flyweight(char)
end
characters.each do |char|
char.display('Arial', 12, 'black')
end
Benefits: Shares the common properties of similar objects, reducing memory consumption and improving efficiency.
Real-World Benefits
Scenario: Rendering Characters in a Graphical Application
Imagine you need to render a large number of characters on the screen with various properties. Using the Flyweight pattern, you can minimize memory usage by sharing the common properties of similar characters.
Without Flyweight Pattern:
class Character
def initialize(char, font, size, color)
@char = char
@font = font
@size = size
@color = color
end
def display
puts "Displaying #{@char} in #{@font} font, size #{@size}, color #{@color}"
end
end
characters = []
('a'..'z').each do |char|
characters << Character.new(char, 'Arial', 12, 'black')
end
characters.each(&:display)
Drawbacks: High memory consumption due to each character object storing its own properties.
With Flyweight Pattern:
class CharacterFlyweight
def initialize(char)
@char = char
end
def display(font, size, color)
puts "Displaying #{@char} in #{font} font, size #{size}, color #{color}"
end
end
class FlyweightFactory
def initialize
@flyweights = {}
end
def get_flyweight(char)
@flyweights[char] ||= CharacterFlyweight.new(char)
end
end
factory = FlyweightFactory.new
characters = []
('a'..'z').each do |char|
characters << factory.get_flyweight(char)
end
characters.each do |char|
char.display('Arial', 12, 'black')
end
Benefits: Minimizes memory usage by sharing the common properties of similar objects.
Conclusion
The Flyweight pattern is a powerful tool for minimizing memory usage by sharing as much data as possible with similar objects. It promotes efficiency and scalability in your code. By using the Flyweight pattern, you can support a large number of fine-grained objects without consuming excessive memory. Incorporate the Flyweight pattern into your design strategies to build more efficient and scalable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!