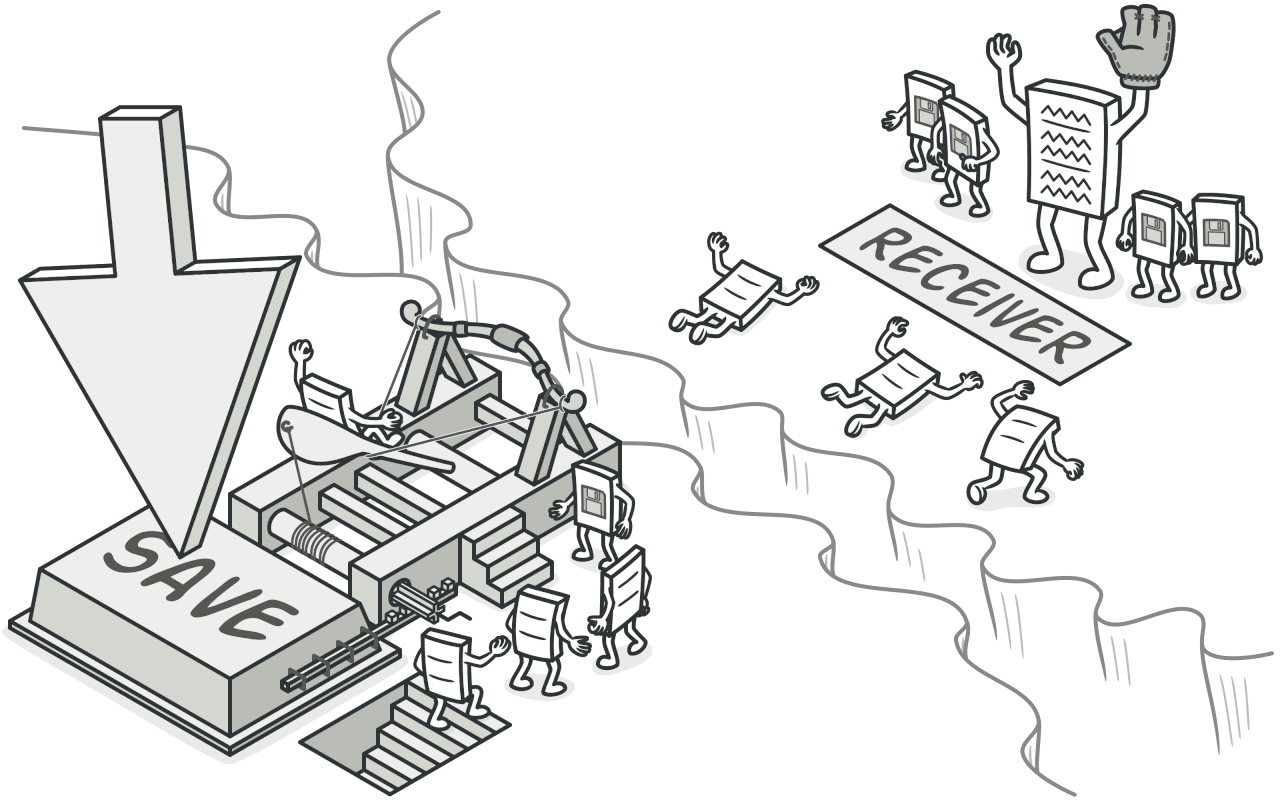
Deep Dive into Behavioral Patterns - The Command Pattern.
Hey software designers! Today, we’re diving into the Command pattern. This pattern is essential for turning a request into a stand-alone object that contains all information about the request. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Command Pattern?
The Command pattern is a behavioral design pattern that turns a request into a stand-alone object that contains all information about the request. This transformation lets you parameterize methods with different requests, delay or queue a request’s execution, and support undoable operations.
Real-World Scenario
Imagine you’re developing a text editor that supports operations like copy, paste, and undo. Each operation can be represented as a command object, allowing you to easily implement undo functionality and manage the history of operations.
The Problem
When managing operations that need to be executed, undone, or queued, hardcoding the logic to handle these operations can lead to a tightly coupled and inflexible codebase. This approach makes it difficult to add or modify operations without changing the existing code.
Without Command Pattern
class TextEditor
def initialize
@text = ''
end
def copy(text)
@clipboard = text
end
def paste
@text += @clipboard
end
def undo
# Implementation for undoing the last operation
end
end
editor = TextEditor.new
editor.copy('Hello')
editor.paste
puts editor.text # Output: Hello
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new operations.
The Solution: Command Pattern
Using the Command pattern, we can encapsulate each operation as a command object, promoting flexibility and scalability.
With Command Pattern
Step 1: Define the Command Interface
class Command
def execute
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def undo
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Commands
class CopyCommand < Command
def initialize(editor, text)
@editor = editor
@text = text
end
def execute
@editor.copy(@text)
end
def undo
@editor.undo_copy
end
end
class PasteCommand < Command
def initialize(editor)
@editor = editor
end
def execute
@editor.paste
end
def undo
@editor.undo_paste
end
end
Step 3: Implement the Receiver
class TextEditor
attr_reader :text
def initialize
@text = ''
@clipboard = ''
end
def copy(text)
@clipboard = text
end
def paste
@text += @clipboard
end
def undo_copy
@clipboard = ''
end
def undo_paste
@text = @text[0...-@clipboard.length]
end
end
Step 4: Implement Client Code
editor = TextEditor.new
copy_command = CopyCommand.new(editor, 'Hello')
paste_command = PasteCommand.new(editor)
copy_command.execute
paste_command.execute
puts editor.text # Output: Hello
paste_command.undo
puts editor.text # Output:
Benefits: Encapsulates each operation as a command object, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Operations
Imagine you need to add a new operation (e.g., Cut). Using the Command pattern, you can easily introduce a new command for the Cut operation without modifying the existing code.
Without Command Pattern:
class TextEditor
def cut(text)
@clipboard = text
@text = @text.gsub(text, '')
end
end
editor = TextEditor.new
editor.cut('Hello')
puts editor.text # Output:
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Command Pattern:
class CutCommand < Command
def initialize(editor, text)
@editor = editor
@text = text
end
def execute
@editor.cut(@text)
end
def undo
@editor.undo_cut
end
end
class TextEditor
def cut(text)
@clipboard = text
@text = @text.gsub(text, '')
end
def undo_cut
@text += @clipboard
end
end
cut_command = CutCommand.new(editor, 'Hello')
cut_command.execute
puts editor.text # Output:
cut_command.undo
puts editor.text # Output: Hello
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Command pattern is a powerful tool for turning a request into a stand-alone object that contains all information about the request. It promotes flexibility, scalability, and maintainability in your code. By using the Command pattern, you can easily manage operations that need to be executed, undone, or queued. Incorporate the Command pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!!