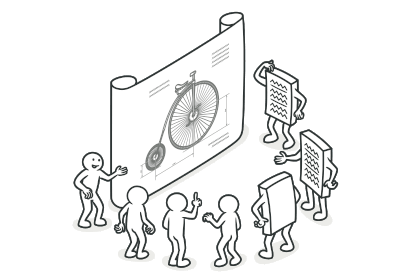
Deep Dive into Behavioral Patterns - The Interpreter Pattern.
Hey software designers! Today, we’re diving into the Interpreter pattern. This pattern is essential for defining a grammatical representation for a language and providing an interpreter to deal with this grammar. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Interpreter Pattern?
The Interpreter pattern is a behavioral design pattern that defines a grammatical representation for a language and provides an interpreter to deal with this grammar. It is useful for implementing specialized languages or for parsing expressions in a language.
Real-World Scenario
Imagine you’re developing a calculator that can evaluate mathematical expressions. Each expression needs to be parsed and evaluated based on its grammar.
The Problem
When dealing with complex expressions or languages, directly interpreting the expressions can lead to a tightly coupled and inflexible codebase. This approach makes it difficult to add or modify the grammar without changing the existing code.
Without Interpreter Pattern
class Calculator
def evaluate(expression)
# Evaluate the expression directly
eval(expression)
end
end
calculator = Calculator.new
puts calculator.evaluate('3 + 5') # Output: 8
puts calculator.evaluate('10 / 2') # Output: 5
Drawbacks: The code is tightly coupled to specific implementations and hard to extend to support new grammar rules.
The Solution: Interpreter Pattern
Using the Interpreter pattern, we can define a grammar for the expressions and provide an interpreter to evaluate them, promoting flexibility and scalability.
With Interpreter Pattern
Step 1: Define the Abstract Expression
class Expression
def interpret
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Terminal and Non-Terminal Expressions
class Number < Expression
def initialize(value)
@value = value
end
def interpret
@value
end
end
class Add < Expression
def initialize(left, right)
@left = left
@right = right
end
def interpret
@left.interpret + @right.interpret
end
end
class Subtract < Expression
def initialize(left, right)
@left = left
@right = right
end
def interpret
@left.interpret - @right.interpret
end
end
Step 3: Implement Client Code
expression = Add.new(Number.new(3), Number.new(5))
puts expression.interpret # Output: 8
expression = Subtract.new(Number.new(10), Number.new(2))
puts expression.interpret # Output: 8
Benefits: Defines a grammatical representation for a language and provides an interpreter to evaluate expressions, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Grammar Rules
Imagine you need to add a new grammar rule (e.g., multiplication). Using the Interpreter pattern, you can easily introduce a new terminal or non-terminal expression without modifying the existing code.
Without Interpreter Pattern:
class Calculator
def evaluate(expression)
# Evaluate the expression directly
eval(expression)
end
end
puts calculator.evaluate('3 * 5') # Output: 15
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Interpreter Pattern:
class Multiply < Expression
def initialize(left, right)
@left = left
@right = right
end
def interpret
@left.interpret * @right.interpret
end
end
expression = Multiply.new(Number.new(3), Number.new(5))
puts expression.interpret # Output: 15
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Interpreter pattern is a powerful tool for defining a grammatical representation for a language and providing an interpreter to evaluate expressions. It promotes flexibility, scalability, and maintainability in your code. By using the Interpreter pattern, you can easily manage and extend the grammar of a language without tightly coupling the code. Incorporate the Interpreter pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!!