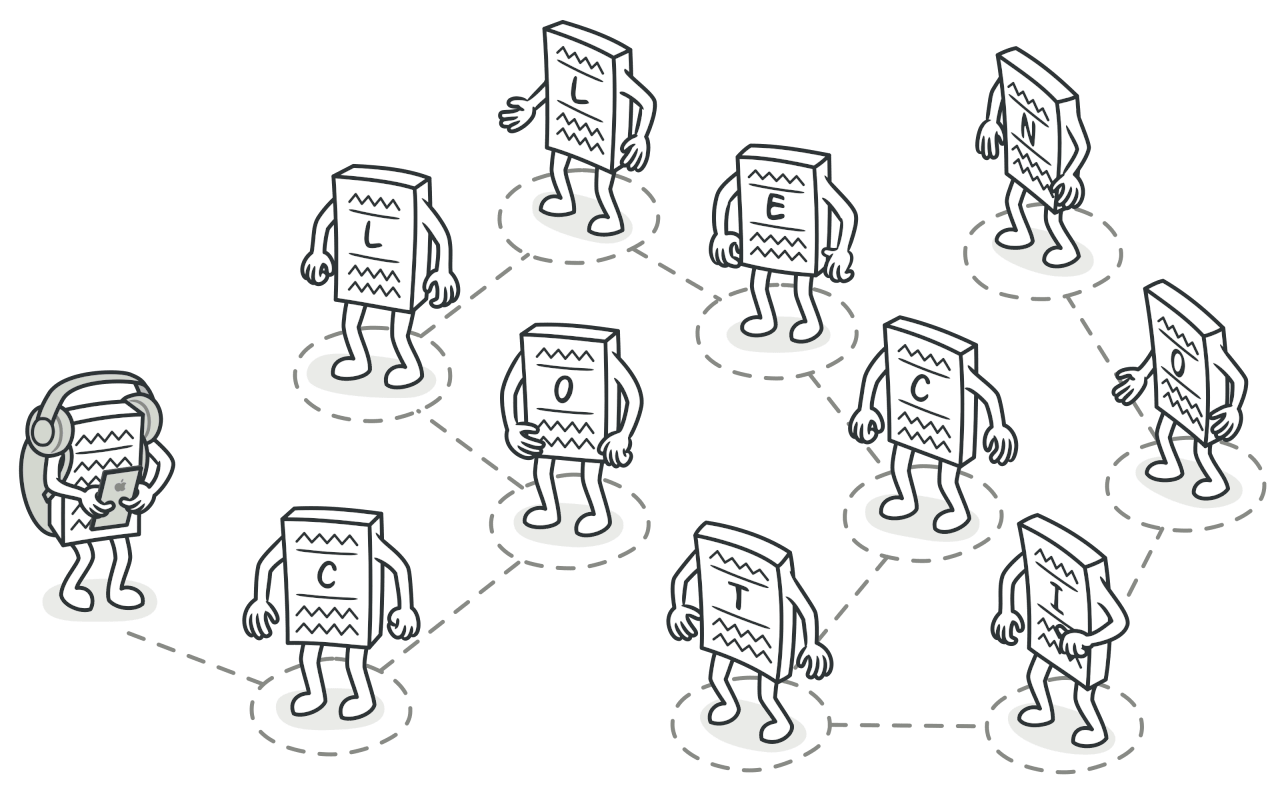
Deep Dive into Behavioral Patterns - The Iterator Pattern.
Hey software designers! Today, we’re diving into the Iterator pattern. This pattern is essential for providing a way to access the elements of an aggregate object sequentially without exposing its underlying representation. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Iterator Pattern?
The Iterator pattern is a behavioral design pattern that provides a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It decouples the traversal of a collection from the collection itself, allowing you to implement different traversal algorithms independently of the collection.
Real-World Scenario
Imagine you’re developing a library management system that needs to iterate over a collection of books. Each book can be accessed and processed without exposing the internal structure of the collection.
The Problem
When dealing with collections, directly accessing the elements can lead to a tightly coupled and inflexible codebase. This approach makes it difficult to change the collection’s implementation without modifying the traversal code.
Without Iterator Pattern
class Book
attr_reader :title
def initialize(title)
@title = title
end
end
class Library
def initialize
@books = []
end
def add_book(book)
@books << book
end
def get_books
@books
end
end
library = Library.new
library.add_book(Book.new('The Catcher in the Rye'))
library.add_book(Book.new('To Kill a Mockingbird'))
library.get_books.each do |book|
puts book.title
end
Drawbacks: The code is tightly coupled to the specific implementation of the collection.
The Solution: Iterator Pattern
Using the Iterator pattern, we can decouple the traversal of the collection from the collection itself, promoting flexibility and scalability.
With Iterator Pattern
Step 1: Define the Iterator Interface
class Iterator
def has_next?
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def next
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Iterators
class BookIterator < Iterator
def initialize(books)
@books = books
@index = 0
end
def has_next?
@index < @books.length
end
def next
book = @books[@index]
@index += 1
book
end
end
Step 3: Define the Aggregate Interface
class Aggregate
def create_iterator
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 4: Create Concrete Aggregates
class Library < Aggregate
def initialize
@books = []
end
def add_book(book)
@books << book
end
def create_iterator
BookIterator.new(@books)
end
end
Step 5: Implement Client Code
library = Library.new
library.add_book(Book.new('The Catcher in the Rye'))
library.add_book(Book.new('To Kill a Mockingbird'))
iterator = library.create_iterator
while iterator.has_next?
book = iterator.next
puts book.title
end
Benefits: Decouples the traversal of a collection from the collection itself, promoting flexibility and scalability.
Real-World Benefits
Scenario: Changing the Collection Implementation
Imagine you need to change the implementation of the collection (e.g., using a different data structure). Using the Iterator pattern, you can easily change the collection’s implementation without modifying the traversal code.
Without Iterator Pattern:
class Library
def initialize
@books = {}
@index = 0
end
def add_book(book)
@books[@index] = book
@index += 1
end
def get_books
@books.values
end
end
library = Library.new
library.add_book(Book.new('The Catcher in the Rye'))
library.add_book(Book.new('To Kill a Mockingbird'))
library.get_books.each do |book|
puts book.title
end
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Iterator Pattern:
class Library < Aggregate
def initialize
@books = {}
@index = 0
end
def add_book(book)
@books[@index] = book
@index += 1
end
def create_iterator
BookIterator.new(@books.values)
end
end
library = Library.new
library.add_book(Book.new('The Catcher in the Rye'))
library.add_book(Book.new('To Kill a Mockingbird'))
iterator = library.create_iterator
while iterator.has_next?
book = iterator.next
puts book.title
end
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Iterator pattern is a powerful tool for providing a way to access the elements of an aggregate object sequentially without exposing its underlying representation. It promotes flexibility, scalability, and maintainability in your code. By using the Iterator pattern, you can easily manage the traversal of collections without tightly coupling the code. Incorporate the Iterator pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!!