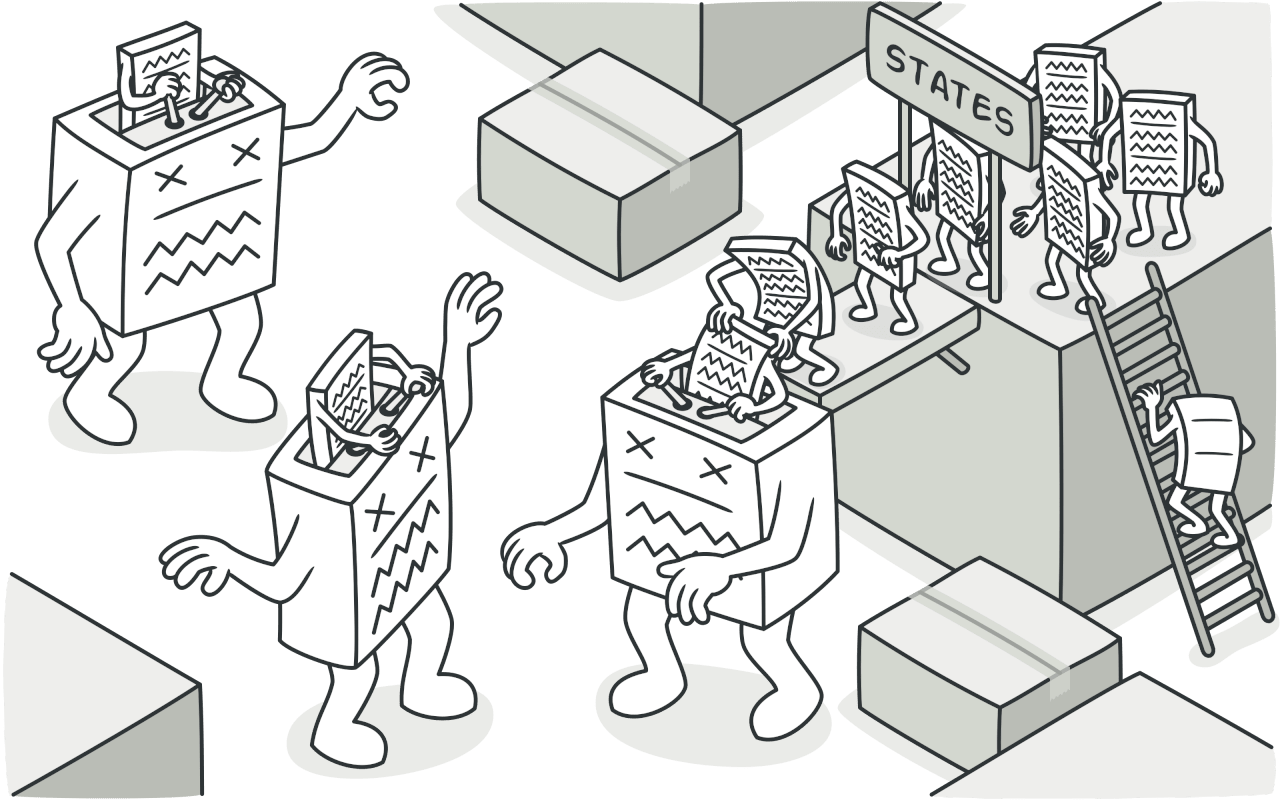
Deep Dive into Behavioral Patterns - The State Pattern.
Hey software designers! Today, we’re diving into the State pattern. This pattern is essential for allowing an object to alter its behavior when its internal state changes. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the State Pattern?
The State pattern is a behavioral design pattern that allows an object to alter its behavior when its internal state changes. The object will appear to change its class. This pattern is particularly useful for implementing state machines and managing states within an application.
Real-World Scenario
Imagine you’re developing a video player application. The player can be in different states like playing, paused, or stopped. Each state has specific behaviors and transitions.
The Problem
When an object needs to change its behavior based on its state, directly implementing state-specific logic within the object can lead to a tightly coupled and inflexible codebase. This approach makes it difficult to add or modify states without changing the existing code.
Without State Pattern
class VideoPlayer
attr_accessor :state
def initialize
@state = 'stopped'
end
def play
if @state == 'stopped'
puts 'Starting playback'
@state = 'playing'
elsif @state == 'paused'
puts 'Resuming playback'
@state = 'playing'
end
end
def pause
if @state == 'playing'
puts 'Pausing playback'
@state = 'paused'
end
end
def stop
if @state == 'playing' || @state == 'paused'
puts 'Stopping playback'
@state = 'stopped'
end
end
end
player = VideoPlayer.new
player.play # Output: Starting playback
player.pause # Output: Pausing playback
player.stop # Output: Stopping playback
Drawbacks: The code is tightly coupled and difficult to extend to support new states.
The Solution: State Pattern
Using the State pattern, we can encapsulate state-specific behavior within separate state classes, promoting flexibility and scalability.
With State Pattern
Step 1: Define the State Interface
class State
def play(player)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def pause(player)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def stop(player)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete States
class PlayingState < State
def play(player)
puts 'Already playing'
end
def pause(player)
puts 'Pausing playback'
player.state = PausedState.new
end
def stop(player)
puts 'Stopping playback'
player.state = StoppedState.new
end
end
class PausedState < State
def play(player)
puts 'Resuming playback'
player.state = PlayingState.new
end
def pause(player)
puts 'Already paused'
end
def stop(player)
puts 'Stopping playback'
player.state = StoppedState.new
end
end
class StoppedState < State
def play(player)
puts 'Starting playback'
player.state = PlayingState.new
end
def pause(player)
puts 'Cannot pause. Player is stopped'
end
def stop(player)
puts 'Already stopped'
end
end
Step 3: Implement the Context
class VideoPlayer
attr_accessor :state
def initialize
@state = StoppedState.new
end
def play
@state.play(self)
end
def pause
@state.pause(self)
end
def stop
@state.stop(self)
end
end
player = VideoPlayer.new
player.play # Output: Starting playback
player.pause # Output: Pausing playback
player.stop # Output: Stopping playback
Benefits: Encapsulates state-specific behavior within separate state classes, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New States
Imagine you need to add a new state (e.g., fast-forwarding). Using the State pattern, you can easily introduce a new state without modifying the existing code.
Without State Pattern:
class VideoPlayer
attr_accessor :state
def initialize
@state = 'stopped'
end
def fast_forward
if @state == 'playing'
puts 'Fast forwarding'
@state = 'fast_forwarding'
end
end
end
player = VideoPlayer.new
player.play
player.fast_forward # Output: Fast forwarding
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With State Pattern:
class FastForwardingState < State
def play(player)
puts 'Cannot play. Player is fast-forwarding'
end
def pause(player)
puts 'Pausing playback'
player.state = PausedState.new
end
def stop(player)
puts 'Stopping playback'
player.state = StoppedState.new
end
end
class PlayingState < State
def fast_forward(player)
puts 'Fast forwarding'
player.state = FastForwardingState.new
end
end
player = VideoPlayer.new
player.play
player.fast_forward # Output: Fast forwarding
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The State pattern is a powerful tool for allowing an object to alter its behavior when its internal state changes. It promotes flexibility, scalability, and maintainability in your code. By using the State pattern, you can easily manage state-specific behavior within your applications without tightly coupling the code. Incorporate the State pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!!