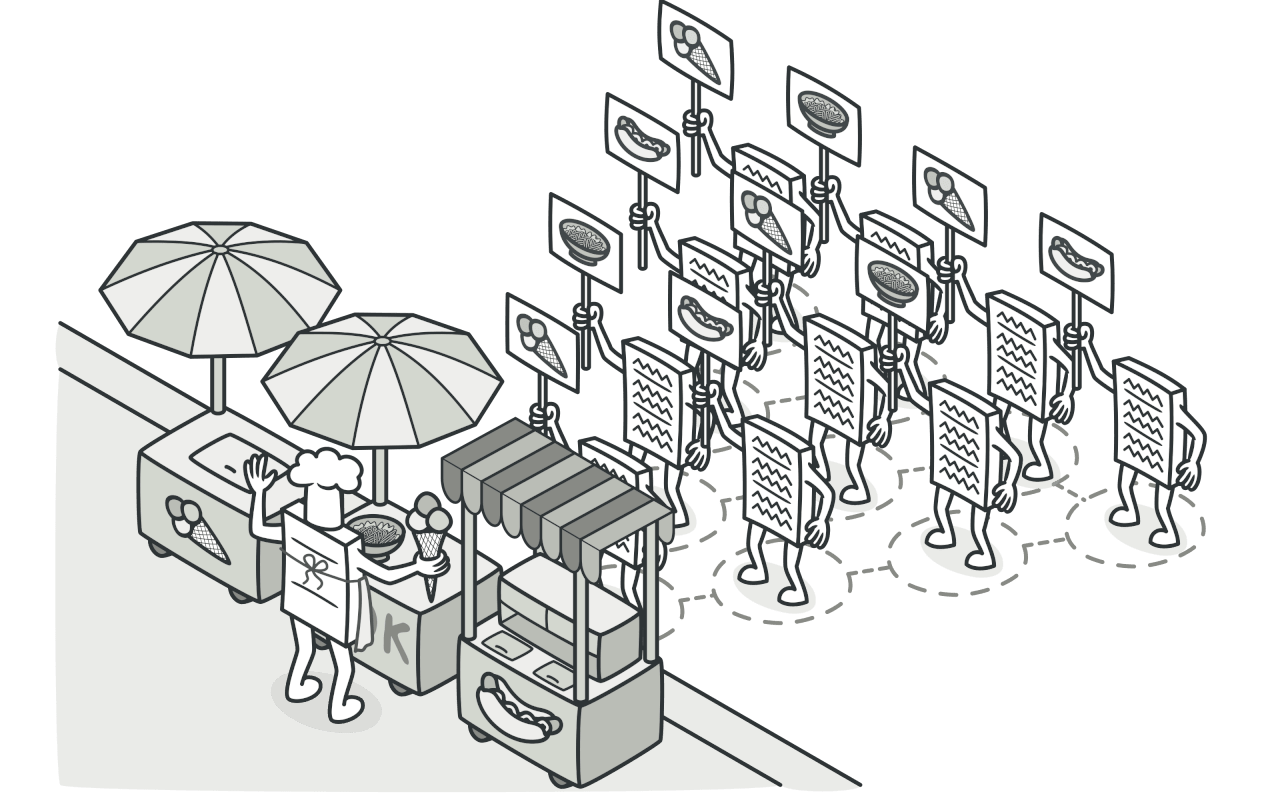
Deep Dive into Behavioral Patterns - The Visitor Pattern.
Hey software designers! Today, we’re diving into the Visitor pattern. This pattern is essential for separating algorithms from the objects on which they operate. Let’s explore its workings, benefits, and real-world applications with detailed examples.
What is the Visitor Pattern?
The Visitor pattern is a behavioral design pattern that allows you to separate algorithms from the objects on which they operate. By using this pattern, you can add new operations to existing object structures without modifying their classes.
Real-World Scenario
Imagine you’re developing a reporting system for a complex object structure in a company (e.g., employees, departments). Each element has specific attributes, but you need to generate various types of reports.
The Problem
When you need to perform operations across a complex object structure, implementing the operations directly within the objects can lead to a tightly coupled and inflexible codebase. This approach makes it difficult to add new operations without modifying the existing code.
Without Visitor Pattern
class Employee
attr_accessor :name, :salary
def initialize(name, salary)
@name = name
@salary = salary
end
def report
"Employee Report: #{@name} - #{@salary}"
end
end
class Department
attr_accessor :name, :employees
def initialize(name)
@name = name
@employees = []
end
def add_employee(employee)
@employees << employee
end
def report
report = "Department Report: #{@name}
"
@employees.each { |e| report += e.report + "
" }
report
end
end
employee = Employee.new('Alice', 50000)
department = Department.new('HR')
department.add_employee(employee)
puts department.report
Drawbacks: The code is tightly coupled and difficult to extend to support new operations.
The Solution: Visitor Pattern
Using the Visitor pattern, we can encapsulate the operations within separate visitor classes, promoting flexibility and scalability.
With Visitor Pattern
Step 1: Define the Element Interface
class Element
def accept(visitor)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 2: Create Concrete Elements
class Employee < Element
attr_accessor :name, :salary
def initialize(name, salary)
@name = name
@salary = salary
end
def accept(visitor)
visitor.visit_employee(self)
end
end
class Department < Element
attr_accessor :name, :employees
def initialize(name)
@name = name
@employees = []
end
def add_employee(employee)
@employees << employee
end
def accept(visitor)
visitor.visit_department(self)
end
end
Step 3: Define the Visitor Interface
class Visitor
def visit_employee(employee)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
def visit_department(department)
raise NotImplementedError, "#{self.class} has not implemented method '#{__method__}'"
end
end
Step 4: Create Concrete Visitors
class ReportVisitor < Visitor
def visit_employee(employee)
"Employee Report: #{employee.name} - #{employee.salary}"
end
def visit_department(department)
report = "Department Report: #{department.name}
"
department.employees.each { |e| report += visit_employee(e) + "
" }
report
end
end
Step 5: Implement the Client Code
employee = Employee.new('Alice', 50000)
department = Department.new('HR')
department.add_employee(employee)
visitor = ReportVisitor.new
puts department.accept(visitor)
Benefits: Encapsulates operations within separate visitor classes, promoting flexibility and scalability.
Real-World Benefits
Scenario: Adding New Operations
Imagine you need to add a new operation (e.g., calculating total salaries). Using the Visitor pattern, you can easily introduce a new visitor class without modifying the existing code.
Without Visitor Pattern:
class Department
def total_salaries
@employees.sum(&:salary)
end
end
puts department.total_salaries
Drawbacks: Tightly coupled code that is difficult to maintain and extend.
With Visitor Pattern:
class SalaryVisitor < Visitor
def visit_employee(employee)
employee.salary
end
def visit_department(department)
department.employees.sum { |e| visit_employee(e) }
end
end
visitor = SalaryVisitor.new
puts department.accept(visitor)
Benefits: Clean, maintainable code with high flexibility and extensibility.
Conclusion
The Visitor pattern is a powerful tool for separating algorithms from the objects on which they operate. It promotes flexibility, scalability, and maintainability in your code. By using the Visitor pattern, you can easily add new operations to existing object structures without modifying their classes. Incorporate the Visitor pattern into your design strategies to build more robust and adaptable software systems.
Stay tuned for more insights into software design principles and patterns.
Thôi Lo Code Đi Kẻo Sếp nạt!!