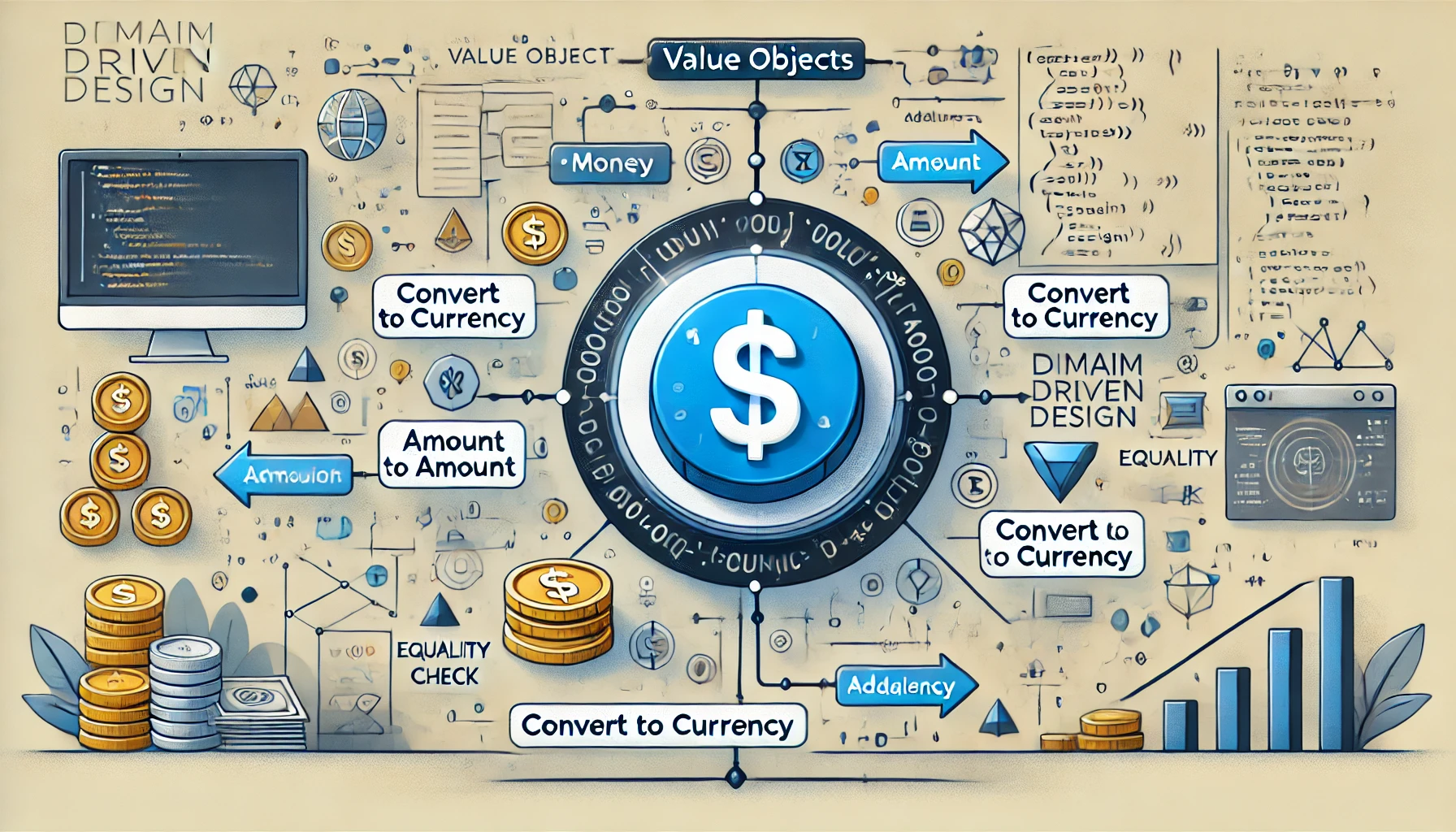
Understanding Value Objects in Domain-Driven Design
Hello, fellow software designers! Today, we’re diving into Value Objects, a key concept in Domain-Driven Design (DDD) that helps make your software more expressive and maintainable. Let’s explore what value objects are, why they matter, and how to use them effectively in your applications.
What Are Value Objects?
A Value Object is a small, immutable object that represents a simple entity like a currency, date, or measurement. Unlike entities, value objects have no unique identity — they are defined entirely by their attributes. If two value objects have the same attributes, they are considered equal.
Why Use Value Objects?
- Encapsulation of Logic: Encapsulate related behavior (like currency conversions or validations) to avoid scattered logic across the codebase.
- Immutability: Make objects immutable to prevent accidental changes, leading to safer, more predictable code.
- Improved Clarity: Communicate the intent of the domain more clearly by using meaningful objects rather than primitive types.
- Reduced Duplication: Centralize domain logic in one place, reducing repetition and making the code easier to maintain.
Real-World Example: Money Handling in E-Commerce
Consider an e-commerce application where you need to handle prices in different currencies. Instead of storing prices as raw numbers, you create a Money
value object:
class Money
attr_reader :amount, :currency
def initialize(amount, currency)
@amount = amount
@currency = currency
end
def convert_to(new_currency)
# Conversion logic here
end
def +(other)
# Addition logic here
end
def ==(other)
amount == other.amount && currency == other.currency
end
end
Benefits of Using Value Objects
- Centralized Logic and Fewer Bugs: All money-related logic is centralized, reducing the risk of errors from duplicated or scattered logic.
- Easier Testing: You can easily unit-test the
Money
class without worrying about the rest of the application. - Better Extensibility: New behavior (like handling a new currency) can be added to the
Money
class without modifying the entire application. - Expressiveness: Code that uses
Money
is more expressive and understandable (price = Money.new(100, 'USD')
) than primitive types (price = 100
).
Challenges and When to Use Value Objects
- Learning Curve: Developers new to Domain-Driven Design may find value objects abstract.
- Overhead: Using many value objects can add perceived overhead, especially in simple applications.
- When to Use: Use value objects when you want to encapsulate logic around simple attributes (like money, dates, measurements) and prevent logic from being scattered across the application.
Conclusion
Value Objects are powerful tools in domain modeling that encapsulate related behavior, reduce duplication, and improve code clarity. By using value objects, you make your code more expressive, maintainable, and aligned with the business domain.
Stay tuned for more insights into software design principles and patterns!
Thôi Lo Code Đi Kẻo Sếp nạt!!